1. What is a Constant ?
Constants are fixed values that do not change during program execution. These fixed values are also called literals.
Using an identifier to represent a constant is called a symbolic constant.
Constants can be of any basic data type, such as integer constants, floating-point constants, character constants, or string literals.
Constants are like regular variables, except that their values cannot be changed after they are defined.
There are different types of constants in C programming.
Constants | Examples |
---|---|
Integer Constant | 10 , 20 , 450 |
Real Number (Floating Point) Constant | 10.3 , 20.2 , 450.6 |
Octal Constant | 021 , 033 , 046 |
Hexadecimal Constant | 0x2a ,0x7b ,0xaa |
Character Constant | 'a' , 'b' ,'x' |
String Constant | "c" , "c program" , "Hello World" |
2. Two Ways to Define Constants
- #define Directive: This method uses the #define preprocessor directive to define a constant. This method does not allocate any memory for the constant and is processed before the compilation process.
- const Keyword: This method uses the const keyword to define a constant. This method allocates memory for the constant and is processed during the compilation process. The const keyword is a type qualifier that specifies that the value of the variable cannot be modified.
2.1 const keyword
The “const” keyword is used to define constants in C programming.
const float PI=3.14;
The value of the PI variable cannot be changed.
Example 2.1.1 Display Constant PI
#include <stdio.h> #include <conio.h> void main() { const float PI = 3.14159; printf("The value of PI is: %f \n", PI); }
example 2.1.1 Results:
The value of PI is: 3.141590 Process returned 30 (0x1E) execution time : 0.887 s Press any key to continue.
Example 2.1.2 Constant Can not be Changed
If you try to change the value of PI, it will lead to a compilation error because PI is defined as a constant variable and its value cannot be modified during the program execution.
#include <stdio.h> #include <conio.h> void main() { const float PI = 3.14159; printf("The value of PI is: %f \n", PI); PI = 10.8; printf("The value of PI is: %f \n", PI); }
Error:Assignment of Ready-Only Variable ‘PI’;
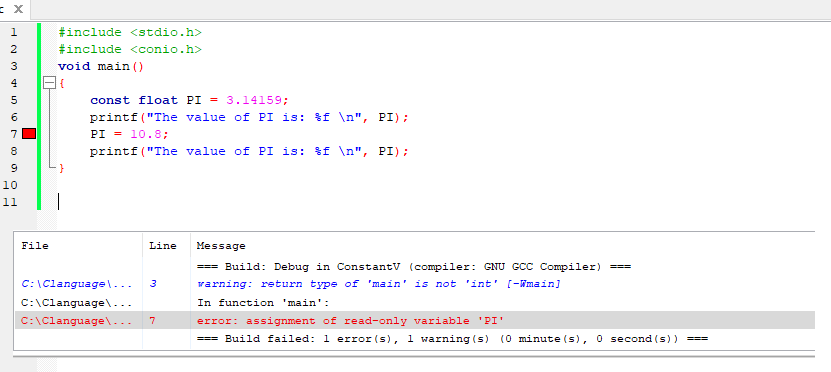
2.2 “#define” Directive
“#define” is a preprocessor directive in C programming language that allows programmers to define constants, macros, and functions before the compilation process. The “#define” directive is used to define a macro that substitutes a specific value or code fragment for every occurrence of an identifier in the source code. These macros can be used to simplify code, make it more readable, or to create constants that are used throughout the program.
Example 2.2.1 Constant Definition Using “#define”
#include <stdio.h> #define PI 3.14 main() { printf("contstant PI = %f",PI); }
Example 2.2.1 Results:
contstant PI = 3.140000 Process returned 0 (0x0) execution time : 0.043 s Press any key to continue.
As a convention, symbol constant identifiers are written in uppercase letters, while variable identifiers are written in lowercase letters, to distinguish between the two.Using an identifier to represent a constant value is called a symbol constant.
Symbol constants are different from variables in that their value cannot be changed within their scope, and they cannot be reassigned a new value.
The advantages of using symbol constants are:
- Clear meaning;
- Achieve “change once, change everywhere” effect.