1. What is C Programming Operators
C language supports a rich set of built-in operators.
An operator is a symbol that operates on a value or a variable. For example: +
is an operator to perform addition.
Operators are symbols that instruct the compiler to perform mathematical, conditional, or logical operations based on the values provided to the operator.
Operands are the values that can be used by any operator. For example, when we say 4 + 5
, the numbers 4
and 5
are operands, while +
is the operator.
Different operators require a different number of operands.
- binary operator, the + operator requires two operands (
5 + 6
), and it is called a binary operator - unary operator, The increment operator “++” only requires one operand (a++), and it is called a unary operator.
- ternary operator, operators that require three operands, known as ternary operators, such as the conditional operator (?:).
2. Types of Operators in C:
C Programming has six types of operators, as follows:
- Arithmetic Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Conditional Operator (?:)
- Miscellaneous Operators
The table below lists all the miscellaneous operators supported by the C language:
Operator | Explanation | Example |
---|---|---|
sizeof() | Returns the size in bytes of a variable or data type. | sizeof(int) will return 4 |
& | Returns the address of a variable. | &a will give the actual address of the variable |
* | Points to a variable. | *a will point to a variable |
. | Accesses members of a structure or union. | mem.age accesses the ‘age’ member in the ‘mem’ structure |
-> | Accesses members of a structure or union through a pointer. | mem->age accesses the ‘age’ member in the ‘mem’ structure using a pointer |
[] | Indexes an element of an array. | arr[0] accesses the first element of the array |
This chapter will only cover arithmetic operators, assignment operators, and the sizeof operator. The topics of relational operators, logical operators, bitwise operators, and miscellaneous operators will be discussed in other chapters.
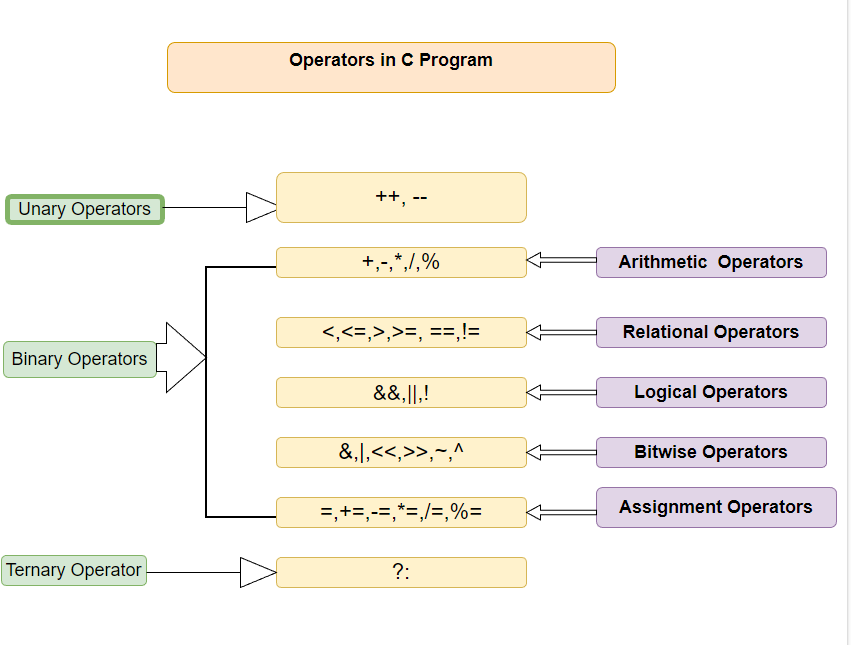
3. Arithmetic Operators
An arithmetic operator performs mathematical operations such as addition, subtraction, multiplication, division etc on numerical values (constants and variables).
The following table shows all the arithmetic operators supported by the C language. Assuming variable A has a value of 18 and variable B has a value of 5:
Operator | Description | Example |
---|---|---|
+ | Adds two numbers | A + B will result in 23 |
– | Subtracts one number from another | A – B will result in 13 |
* | Multiplies two numbers | A * B will result in 90 |
/ | Divides the numerator by the denominator | A / B will result in 3.6 |
% | Modulus operator, returns the remainder after division | B % A will result in 3 |
++ | Increment operator, increases the integer value by 1 | A++ will result in 19 |
— | Decrement operator, decreases the integer value by 1 | A– will result in 17 |
Example 3.1) Application of arithmetic operators.
#include <stdio.h> int main() { int A=18; int B=5; printf("A=%d\n", A); printf("B=%d\n",B); printf("A+B=%d\n",A+B); printf("A-B=%d\n",A-B); printf("A*B=%d\n",A*B); printf("A/B=%d\n",A/B); int C = A % B ; printf("A Remainder B=%d\n",C); A++; // to increase the value of a variable by 1 printf("A++=%d\n",A); B--; // to decrease the value of a variable by 1 printf("B--=%d\n",B); return 0; }
Results:
A=18
B=5
A+B=23
A-B=13
A*B=90
A/B=3
A Reminder B=3
A++=19
B--=4
Process returned 0 (0x0) execution time : 0.039 s
Press any key to continue.
3.1 C Increment and Decrement Operators
There are two ways to perform increment or decrement operations on a variable in C:
C programming has two operators increment ++
and decrement --
to change the value of an operand (constant or variable) by 1.
Increment ++
increases the value by 1 whereas decrement --
decreases the value by 1.
Post-increment:
variable++; // Represents incrementing the variable after its current value is used;
Pre-increment:
++variable; // Represents incrementing the variable before its value is used;
Post-decrement:
variable–; // Represents decrementing the variable after its current value is used;
Pre-decrement:
–variable; // Represents decrementing the variable before its value is used.
example 3.2 ) The increment and decrement operators
#include <stdio.h> int main() { int ii; ii=10; printf("ii=%d\n",ii); printf("ii++=%d\n",ii++); // Post-increment printf("ii=%d\n\n",ii); ii=10; printf("ii=%d\n",ii); printf("++ii=%d\n",++ii); // Pre-increment printf("ii=%d\n\n",ii); ii=10; printf("ii=%d\n",ii); printf("--ii=%d\n",--ii); // Pre-decrement printf("ii=%d\n\n",ii); ii=10; printf("ii=%d\n",ii); printf("ii--=%d\n",--ii); // Post-decrement printf("ii=%d\n\n",ii); return 0; }
results:
ii=10
ii++=10
ii=11
ii=10
++ii=11
ii=11
ii=10
--ii=9
ii=9
ii=10
ii--=9
ii=9
Process returned 0 (0x0) execution time : 0.957 s
Press any key to continue.
4. C Assignment Operators
An assignment operator is used for assigning a value to a variable. The most common assignment operator is =
Operator | Description | Example |
= | Simple assignment operator. Assigns the right operand to the left operand. | int x = 10; int y; y = x; // y is assigned the value of x ( 10 ) |
+= | Addition assignment operator. Adds the right operand to the left operand and assigns the result to the left operand. | int a = 5; a += 3; // a is incremented by 3 |
-= | Subtraction assignment operator. Subtracts the right operand from the left operand and assigns the result to the left operand. | int b = 8; b -= 4; // b is decremented by 4 |
*= | Multiplication assignment operator. Multiplies the left operand by the right operand and assigns the result to the left operand. | int c = 3; c *= 2; // c is multiplied by 2 |
/= | Division assignment operator. Divides the left operand by the right operand and assigns the result to the left operand. | int d = 12; d /= 4; // d is divided by 4 |
%= | Modulus assignment operator. Calculates the modulus of the two operands and assigns the result to the left operand. Not applicable to floating-point numbers. | int e = 10; e %= 3; // e is assigned the remainder of e divided by 3 |
Assignment operators support basic data types in the C language, including char, int, and double. However, strings (character arrays) cannot be directly assigned using assignment operators.
Example 4.1 Assignment operators
#include <stdio.h> int main() { int C=0; int A=21; printf("C=0; A=21\n"); C=A; printf("C=A; C=%d\n",C); C+=A; // C=C+A; printf("C+=A,C=%d\n",C); C-=A; // C=C-A; printf("C-=A,C=%d\n",C); C*=A; // C=C*A; printf("C*=A,C=%d\n",C); C/=A; // C=C/A; printf("C/=A,C=%d\n",C); C=200; printf("C=200\n"); C%=A; // C=C%A; printf("C%=A,C=%d\n",C); return 0; }
results:
C=0; A=21
C=A; C=21
C+=A,C=42
C-=A,C=21
C*=A,C=441
C/=A,C=21
C=200
C=A,C=11
Process returned 0 (0x0) execution time : 1.185 s
Press any key to continue.
5. The sizeof() Operator
sizeof() is a unary operator in the C language, like other operators such as ++ and –, it is not a function.
The sizeof() operator provides the storage size of its operand in bytes. The operand can be an expression or a type name enclosed in parentheses.
The storage size of the operand is determined by the type of the operand.
The result of sizeof of different data types (which may vary on different platforms) :
sizeof(char) = 1; sizeof(unsigned char) = 1; sizeof(signed char) = 1; sizeof(int) = 4; sizeof(unsigned int) = 4; sizeof(short int) = 2; sizeof(unsigned short) = 2; sizeof(long int) = 4; sizeof(unsigned long) = 4; sizeof(float) = 4; sizeof(double) = 8; sizeof(long double) = 12;
example 5.1 The sizeof() Operator
#include <stdio.h> int main() { int a; float b; double c; char d; printf("Size of int=%lu bytes\n",sizeof(a)); printf("Size of float=%lu bytes\n",sizeof(b)); printf("Size of double=%lu bytes\n",sizeof(c)); printf("Size of char=%lu byte\n",sizeof(d)); return 0; }
Results:
Size of int = 4 bytes
Size of float = 4 bytes
Size of double = 8 bytes
Size of char = 1 byte
6. Relational operator
Relational operators, also known as comparison operators, are used in C programming to establish relationships between values. Similar to mathematical expressions like 10 > 9 or 1 < x < 5, relational operators evaluate a condition and produce a result that can be either true or false. For example, the statement “1 > 2” is a false condition.
In computer programming, these relational operations are also supported, but the result is binary, meaning it can only be either true or false. When a condition is evaluated and found to be true, it is referred to as “true,” and when it is false, it is referred to as “false.” Each condition evaluation can only yield one of these two outcomes and cannot have other possibilities. Additionally, the same condition will always produce the same result, regardless of the context.
Relational operators allow us to compare values, such as checking if one value is greater than or equal to another, less than or equal to another, or equal to another. These comparisons are fundamental for decision-making and control flow in programming.
Operator | Description | Example(a and b, a = 10, b = 11) |
== |
Checks if the values of two operands are equal, and if so, the condition becomes true. | a == b, false |
!= |
Checks if the values of two operands are not equal, and if so, the condition becomes true. | a != b, true |
> |
Checks if the value of the left operand is greater than the value of the right operand, and if so, the condition becomes true | a > b, false |
< |
Checks if the value of the left operand is less than the value of the right operand, and if so, the condition becomes true | a < b, true |
>= |
Checks if the value of the left operand is greater than or equal to the value of the right operand, and if so, the condition becomes true. | a >= b, false |
<= |
Checks if the value of the left operand is less than or equal to the value of the right operand, and if so, the condition becomes true. | a <= b, true |
Example 6. 1. Relational Operators
#include <stdio.h> int main() { int a = 10, b = 20, result; // Equal result = (a==b); printf("(a == b) = %d \n",result); // less than result = (a<b); printf("(a < b) = %d \n",result); // greater than result = (a>b); printf("(a > b) = %d \n",result); // less than equal to result = (a<=b); printf("(a <= b) = %d \n",result); return 0; }
In C programming language, true represents any value except zero. False is represented by zero.
7. Logical operators
Logical operators in C are used to perform logical operations on boolean values (true or false). They evaluate the conditions and return a boolean result. C language supports three logical operators: &&
(logical AND), ||
(logical OR), and !
(logical NOT).
7.1. Logical AND (&&
)
Description: The logical AND operator returns true if both of its operands are true. Otherwise, it returns false.
Example:
int a = 5; int b = 10; int c = 0; int result1, result2; result1 = (a > 0 && b > 0); // Evaluates to true (1) because both 'a' and 'b' are positive result2 = (a > 0 && c > 0); // Evaluates to false (0) because 'c' is not positive printf("Result1: %d\n", result1); // Output: Result1: 1 printf("Result2: %d\n", result2); // Output: Result2: 0
7.2. Logical OR (||)
Description: The logical OR operator returns true if at least one of its operands is true. If both operands are false, it returns false.
Example:
int a = 5; int b = 10; int c = 0; int result1, result2; result1 = (a > 0 || b > 0); // Evaluates to true (1) because at least one of 'a' and 'b' is positive result2 = (a > 0 || c > 0); // Evaluates to true (1) because 'a' is positive printf("Result1: %d\n", result1); // Output: Result1: 1 printf("Result2: %d\n", result2); // Output: Result2: 1
7.3. Logical NOT (!)
Description: The logical NOT operator reverses the logical state of its operand. If the operand is true, it returns false. If the operand is false, it returns true.
int a = 5; int b = 0; int result1, result2; result1 = !b; // Evaluates to true (1) because 'b' is zero result2 = !(a > 10); // Evaluates to true (1) because 'a' is not greater than 10 printf("Result1: %d\n", result1); // Output: Result1: 1 printf("Result2: %d\n", result2); // Output: Result2: 1
In the examples provided, the results of the logical operations are assigned to variables result1 and result2 for clarity. The values of these variables are then printed using printf statements. The results demonstrate the logical behavior of the operators where true is represented by 1 and false is represented by 0.
Operator | Description | Example (a and b, a = 1, b = 0) |
&& |
logical AND operator. It returns true if both operands are non-zero | a && b, result: false |
|| |
logical OR operator. It returns true if either of the operands are non-zero | a || b, result: true |
! |
logical NOT operator. is used to invert the logical state of an operand | ! a, result:0 |
Example 7.3.1) Logical Operators
#include <stdio.h> int main() { int a = 1, b = 0, result; // And result = (a && b); printf("a && b = %d \n",result); // Or result = (a || b); printf("a || b = %d \n",result); // Not result = !a; printf("!a = %d \n",result); return 0; }
Results:
a && b = 0
a || b = 1
!a = 0
Process returned 0 (0x0) execution time : 0.032 s
Press any key to continue.
Example 7.3.2) Logical Operators
// Working of logical operators #include <stdio.h> int main() { int a = 5, b = 5, c = 10, result; result = (a == b) && (c > b); printf("(a == b) && (c > b) is %d \n", result); result = (a == b) && (c < b); printf("(a == b) && (c < b) is %d \n", result); result = (a == b) || (c < b); printf("(a == b) || (c < b) is %d \n", result); result = (a != b) || (c < b); printf("(a != b) || (c < b) is %d \n", result); result = !(a != b); printf("!(a != b) is %d \n", result); result = !(a == b); printf("!(a == b) is %d \n", result); return 0; }
Results:
(a == b) && (c > b) is 1
(a == b) && (c < b) is 0
(a == b) || (c < b) is 1
(a != b) || (c< b) is 0
!(a != b) is 1
!(a == b) is 0
8. C Program : Bitwise Operators
During computation, mathematical operations like: addition, subtraction, multiplication, division, etc are converted to bit-level which makes processing faster and saves power.
8.1. What Are Bitwise Operators ?
Bitwise operators are used in C programming to perform bit-level operations.
Bitwise operators are not applicable to operands of type float, double, long double, or void. Bitwise operators are specifically designed to operate on integral data types, such as int, char, and long, by manipulating the individual bits of the operands.
Attempting to use bitwise operators on float, double, long double, or void operands will result in a compilation error or undefined behavior. These data types do not represent the data at the bit level, so bitwise operations are not meaningful or well-defined for them.
If you need to perform bitwise operations, make sure to use appropriate integer data types instead of floating-point or void types.
&
Bitwise AND operator. Performs a logical AND operation on each corresponding bit of the operands. Returns 1 if both bits are 1, otherwise returns 0.
|
Bitwise OR operator. Performs a logical OR operation on each corresponding bit of the operands. Returns 1 if at least one of the bits is 1, otherwise returns 0.
^
Bitwise XOR (exclusive OR) operator. Performs a logical XOR operation on each corresponding bit of the operands. Returns 1 if the bits are different, otherwise returns 0.
~
Bitwise NOT operator. Flips each bit of the operand, changing 0 to 1 and 1 to 0. Returns the complement of the operand.
<<
Left shift operator. Shifts the bits of the left operand to the left by a specified number of positions. The empty positions are filled with zeros.
>>
Right shift operator. Shifts the bits of the left operand to the right by a specified number of positions. The empty positions are filled based on the sign of the left operand.
Explanation:
(1) Except for the bitwise NOT operator (~), all other bitwise operators are binary operators, which means they require operands on both sides.
(2) The operands must be of integer or character types. They cannot be floating-point numbers.
(3) Bitwise operators operate on the binary representation of the data, specifically on the two’s complement representation. The bitwise operations are performed on each corresponding bit of the operands, allowing you to manipulate individual bits.
These bitwise operators are commonly used in tasks such as manipulating individual bits, setting or clearing specific flags or bits, performing bitwise operations on bit masks, and optimizing memory usage. It’s important to remember that bitwise operators operate on the binary representation of the data, and the operands must be integer or character types.
8.2. Truth Table Showing the Results of Bitwise Operators
Truth Table of &, |, ^
a | b | a & b | a | b | a ^ b |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 0 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
8.3. Left Shift Operator and Right Shift Operator
8.3.1 example
a = 00010000 b = 2 a << b = 01000000 a >> b = 00000100
In the case of a << b, the value 00010000 is shifted to the left by 2 bits, and additional zeros are added on the right. Therefore, the value becomes 01000000. For a >> b, the value is shifted 2 bits to the right. Two zeros are removed from the right, and two zeros are added on the left. Therefore, the value becomes 00000100.
It’s important to note that shifting does not work like rotation, which means that the shifted bits are not added to the other end. The value of the shifted bits is lost.
8.4.~
Bitwise NOT operator
Let’s understand the bitwise NOT operator through an example:
unsigned int a = 44; // binary: 00101100 unsigned int result = ~a; // binary: 11010011
In this example, we have the variable a with a value of 44, which is represented in binary as 00101100. Applying the bitwise NOT operator (~) to a flips each bit, changing each 1 to 0 and each 0 to 1. As a result, we obtain 11010011 in binary.
So, the bitwise NOT operator complements each bit of the operand, effectively changing all 1s to 0s and all 0s to 1s. It is also known as the one’s complement operator.
Example 8.4.1 Bitwise NOT operator and Left Shift Operator and Right Shift Operator
#include <stdio.h> int main() { int a = 0001000, b = 2, result; printf("a=%d | b=%d\n",a,b); // << result = a<<b; printf("a << b = %d \n",result); // >> result = a>>b; printf("a >> b = %d \n",result); return 0; }
Results:
a=512 | b=2
a << b = 2048
a >> b = 128
Example 8.4.2 Bitwise operators
// C Program to demonstrate use of bitwise operators #include <stdio.h> int main() { // a = 5(00000101), b = 9(00001001) unsigned char a = 5, b = 9; // The result is 00000001 printf("a = %d, b = %d\n", a, b); printf("a&b = %d\n", a & b); // The result is 00001101 printf("a|b = %d\n", a | b); // The result is 00001100 printf("a^b = %d\n", a ^ b); // The result is 11111010 printf("~a = %d\n", a = ~a); // The result is 00010010 printf("b<<1 = %d\n", b << 1); // The result is 00000100 printf("b>>1 = %d\n", b >> 1); return 0; }
Results:
a = 5, b = 9 a&b = 1 a|b = 13 a^b = 12 ~a = 250 b<<1 = 18 b>>1 = 4
8.5. ^
Bitwise XOR (exclusive OR) operator.
The bitwise XOR (exclusive OR) operator is denoted by the symbol ^
and is used to perform a bitwise operation on two operands.
From a technical standpoint, the bitwise XOR operator is the most useful operator. It is used in many problems.
A simple example is “Given an array of numbers where all elements appear even times except for one number that appears odd times, find the number that appears odd times.”
This problem can be effectively solved by performing XOR operation on all the numbers.
It compares the corresponding bits of the operands and produces a new value where each bit is set to 1 if the two corresponding bits are different (one bit is 1 and the other is 0), and set to 0 if the two corresponding bits are the same (both 0 or both 1).
Let’s illustrate the bitwise XOR operator with an example:
unsigned int a = 10; // binary: 00001010 unsigned int b = 6; // binary: 00000110 unsigned int result = a ^ b; // binary: 00001100
In this example, we have two variables a
and b
with values 10 and 6, respectively. When we apply the bitwise XOR operator (^
) to a
and b
, it compares the corresponding bits of a
and b
and produces a new value result
where each bit is set to 1 if the two corresponding bits are different, and set to 0 if the two corresponding bits are the same.
Here’s the breakdown of the bitwise XOR operation:
a: 00001010
b: 00000110
----------------
result: 00001100
So, the resulting value of result
is 00001100
in binary, which is equal to 12 in decimal. The bitwise XOR operator can be used for various purposes, such as flipping specific bits, swapping values, or performing encryption algorithms.
Example 8.5.1 Bitwise XOR Operator – Find the Number That Only Show up odd Times
#include <stdio.h> // Function to return the only odd // occurring element int findOdd(int arr[], int n) { int res = 0, i; for (i = 0; i < n; i++) res ^= arr[i]; return res; } int main(void) { int arr[] = { 12, 12, 14, 90, 14, 14, 14 }; printf("sizeof arr: %d\n", sizeof(arr)); printf("sizeof arr[0]:%d\n", sizeof(arr[0])); int n = sizeof(arr) / sizeof(arr[0]); printf("The odd occurring element is %d ", findOdd(arr, n)); return 0; }
Results:
sizeof arr: 28
sizeof arr[0]:4
The odd occurring element is 90