the do...while
loop is another type of loop construct that is similar to the while
loop. The key difference is that the do...while
loop guarantees that the loop body is executed at least once, as the condition is checked after the execution of the loop body.
Diagram of do…while loop is as follow:
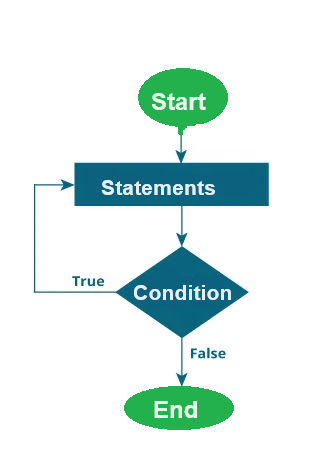
Syntax
do { Statements // code to be executed } while (condition);
Here’s a breakdown of the syntax:
do
: Keyword that introduces the start of thedo...while
loop.{}
: Curly braces that enclose the block of code to be executed in the loop.while (condition)
: The condition that is checked after the execution of the loop body. If this condition is true, the loop will continue; otherwise, it will terminate.- ; : while (condition) must be ended with a Semicolon ;.
Example 1) Calculating the sum of numbers from 1 to 100 using do…while loop
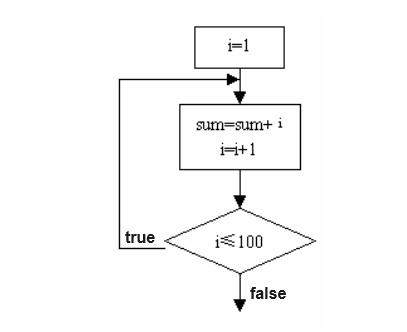
main() { int i,sum=0; i=1; do { sum=sum+i; i++; } while(i<=100); printf("%d\n",sum); }
Example 2) Printing even numbers within 20.
#include<stdio.h> #include<conio.h> int main() { int num=1; //initializing the variable do //do-while loop { printf("%d\n",2*num); num++; //incrementing operation }while(num<=10); return 0; }
Results:
In the above example, we used the do-while loop statement to print the table of even numbers. Let’s take a look at how the program works.
How does the above program work?
- Firstly, we initialize the variable ‘num’ to 1. Then, we have written a do-while loop;
- In each iteration of the loop, we have a print function that prints the value of num multiplied by 2;
- After each increment, the value of num increases by 1, and the result of multiplying it by 2 is printed on the screen;
- When the value of num becomes 10, the loop terminates, and immediately after the loop, one statement is executed.
Example 3) Adding numbers until the user enters zero
#include <stdio.h> int main() { double number, sum = 0; // the body of the loop is executed at least once do { printf("Enter a number: "); scanf("%lf", &number); sum += number; } while(number != 0.0); printf("Sum = %.2lf",sum); return 0; }
we use a do...while
loop to prompt the user to input a number. The loop continues as long as the input number is not 0.
The do...while
loop ensures that it executes at least once because the condition is not checked until after the first iteration. The condition is checked only after the first iteration.
Result:
Enter a number: 1.5 Enter a number: 2.4 Enter a number: -3.4 Enter a number: 4.2 Enter a number: 0 Sum = 4.70
Therefore, if the first input is a non-zero number, that number is added to the sum
variable, and the loop continues to the next iteration. This process is repeated until the user inputs 0.
However, if the first input is 0, the loop won’t go through a second iteration, and sum
remains 0.0.
Outside the loop, we print the value of sum
.
Example 4) Printing numbers from 1 to 5 using do…while loop
#include <stdio.h> int main() { int i = 1; do { printf("%d ", i); i++; } while (i <= 5); return 0; }
Results:
1 2 3 4 5
Explanation:
- The loop initializes
i
to 1. - The
do
block prints the value ofi
. - The loop increments
i
. - The loop checks the condition
i <= 5
. If true, the loop continues; otherwise, it terminates. - The loop continues this process until
i
becomes 6, at which point the condition becomes false, and the loop terminates.