1.What is while Loop Statement ?
The while
loop is a control flow statement that allows a block of code to be repeatedly executed as long as a given condition is true.
Syntex
while (condition) { statements; // Code to be executed repeatedly as long as the condition is true }
2. How while Loop Works?
Here’s how it works step by step:
- Condition Check: The loop begins with the evaluation of a specified condition. If the condition is true, the code inside the loop is executed. If the condition is false initially, the code inside the loop is skipped, and the program moves to the next statement after the
while
loop.while (condition) { // Code inside the loop }
- Execution of Code Block: If the condition is true, the code block inside the
while
loop is executed. This block can contain one or more statements. - Condition Re-evaluation: After executing the code block, the condition is re-evaluated. If the condition is still true, the loop repeats, and the code block is executed again. If the condition is false, the loop is terminated, and the program continues with the statement following the
while
loop. - Iteration: Steps 2 and 3 are repeated in each iteration of the loop until the condition becomes false.
while loop works as following diagram
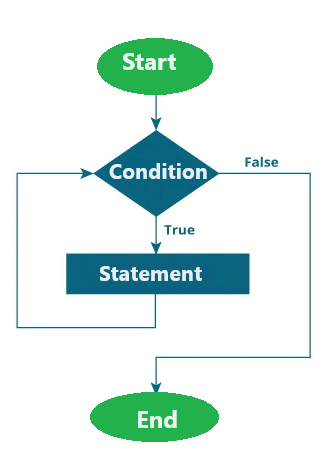
3. Examples
Example 3.1) a while
loop that prints numbers from 1 to 5
#include <stdio.h> int main() { int i = 1; while (i <= 5) { printf("%d ", i); i++; } return 0; }
Result:
1 2 3 4 5
Explanation:
- We initialize a variable
i
with the value 1. - The
while
loop continues to execute as long asi
is less than or equal to 5. - In each iteration, the value of
i
is printed, andi
is incremented by 1 (i++
). - The loop terminates when
i
becomes greater than 5.
Example 3.2 ) To calculate the sum from 1 to 100 using a while
loop
algorithm diagram for the sum from 1 to 100
code:
main() { int i=1,sum=0; while(i<=100) { sum=sum+i; i++; } printf("%d\n",sum); }
This program initializes a variable i to 1 and a variable sum
to 0. Then, it utilizes a while
loop to continually add the value of i to sum
while incrementing i until i exceeds 100.
The output should be the sum from 1 to 100.
Results:
Example 3.3) Print integers from 1 to 10
#include<stdio.h> #include<conio.h> int main() { int num=1; //initializing the variable while(num<=10) //while loop with condition { printf("%d\n",num); num++; //incrementing operation } return 0; }
results:
Example3.3 explanation
Initialize a variable named “num” with a value of 1. We are going to print from 1 to 10, so the variable is initialized with the value 1.
In the while
loop, a condition is provided (num <= 10
), which means the loop will execute the body until the value of num
becomes 10. After that, the loop will terminate, and control will move outside the loop.
In the body of the loop, a print function is used to print the number, along with an increment operation that increases the value each time the loop is executed. The initial value of num
is 1, which becomes 2 after execution and will be 3 in the next iteration.
This process continues until the value becomes 10, at which point it is printed to the console, and the loop terminates.
The %d
is used for formatting, substituting the subsequent integer. \n
is used for formatting purposes, starting a new line.
If changed to \t
(tab), it would print the result like this:
4. Summary
- Initialization: Before entering the loop, initialize the loop control variable.
- Condition: The loop executes as long as the specified condition evaluates to true.
- Execution: The code block inside the while loop is executed repeatedly while the condition is true.
- Increment/Decrement: Inside the loop, update the loop control variable to eventually make the condition false and exit the loop. Failure to do this may result in an infinite loop.
- Entry-controlled: The while loop is an entry-controlled loop, as the condition is checked before entering the loop body.
- Pre-check Loop: The condition is evaluated before the execution of the loop body. If the condition is false initially, the loop body is not executed at all.
- Termination: The loop terminates when the condition becomes false.
- Use Cases: While loops are suitable when the number of iterations is not known beforehand and depends on a certain condition.