In C programming, scanf()
is one of the commonly used function to take input from the user. The scanf()
function reads formatted input from the standard input such as keyboards.
1. Variable Name, Variable Value, and Variable Address
When we declare a variable, the compiler assigns it a memory address. The relationship between the variable name, variable value, and variable address is illustrated in the following diagram:
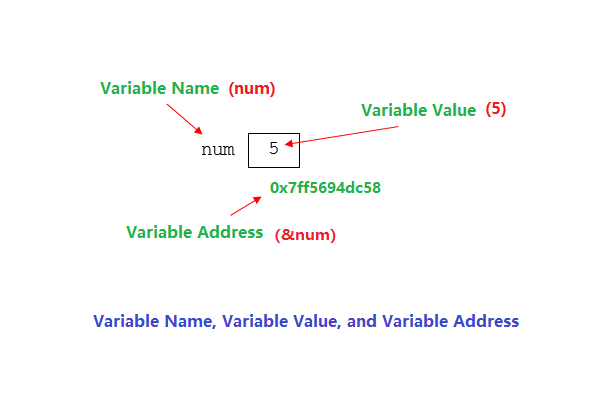
2. scanf() Syntax and Usage
The scanf()
function in C is used to read input from the standard input stream (usually the keyboard) and store it into variables. The scanf()
function reads data from the keyboard and stores it in the memory addresses of variables. It allows the program to interactively accept input from the user during runtime.
The scanf()
function uses format specifiers to determine the type and format of the input to be read. It takes two main arguments: the format string and the addresses of the variables where the input values should be stored.
The general syntax of the scanf()
function is as follows:
scanf(format_string, &variable1, &variable2, ...);
Here, format_string
is a string that contains format specifiers corresponding to the data types of the variables. The format specifiers start with the %
symbol and are followed by a character that represents the data type to be read. The &
operator is used to obtain the memory addresses of the variables.
For example, to read an integer and a floating-point number from the user, you could use the following scanf()
statement:
int num;
float value;
scanf("%d %f", &num, &value);
In this case, the format string "%d %f"
specifies that an integer should be read first, followed by a floating-point number. The values entered by the user will be stored in the num
and value
variables, respectively.
Format Specifiers for I/O
Here are some commonly used format specifiers in C:
%d
– Used for formatting and displaying integers (signed decimal).%f
– Used for formatting and displaying floating-point numbers (decimal notation).%c
– Used for formatting and displaying a single character.%s
– Used for formatting and displaying strings (arrays of characters).%x
– Used for formatting and displaying integers in hexadecimal (lowercase).%X
– Used for formatting and displaying integers in hexadecimal (uppercase).%o
– Used for formatting and displaying integers in octal (base 8).%u
– Used for formatting and displaying unsigned integers (decimal).%e
– Used for formatting and displaying floating-point numbers in scientific notation (lowercase).%E
– Used for formatting and displaying floating-point numbers in scientific notation (uppercase).%g
– Used for formatting and displaying floating-point numbers in either decimal or scientific notation, depending on the value (lowercase).%G
– Used for formatting and displaying floating-point numbers in either decimal or scientific notation, depending on the value (uppercase).%%
– Used to print a literal percent sign%
.
Here’s a list of commonly used C data types and their format specifiers.
Data Type | Format Specifier |
---|---|
int |
%d |
char |
%c |
float |
%f |
double |
%lf |
short int |
%hd |
unsigned int |
%u |
long int |
%li |
long long int |
%lli |
unsigned long int |
%lu |
unsigned long long int |
%llu |
signed char |
%c |
unsigned char |
%c |
long double |
%Lf |
The scanf()
function returns the number of input items successfully matched and assigned. It can be used to check if the input was successfully read.
It’s important to note that when reading strings with scanf()
, you should use the %s
format specifier and provide a buffer size to avoid buffer overflow vulnerabilities.
Additionally, error handling and validation should be implemented to handle unexpected user input and ensure the program behaves correctly.
The scanf()
function converts input strings into integers, floating-point numbers, characters, and strings, while printf()
does the opposite by converting integers, floating-point numbers, characters, and strings into text displayed on the screen.
Similar to printf()
, scanf()
also requires a format string and an argument list. The format string in scanf()
indicates the desired data type of the character input stream.
The main difference between the two functions lies in the argument list. The printf()
function uses variables, constants, and expressions, while the scanf()
function uses pointers to variables.
Example 2.1) Reads Three Integers From the User and Outputs Them (Standard )
#include <stdio.h>
int main() {
int num1, num2, num3;
printf("Enter three integers: ");
scanf("%d %d %d", &num1, &num2, &num3);
printf("You entered: %d, %d, %d\n", num1, num2, num3);
return 0;
}
Example 2.2) Reads Three Integers From the User and Outputs Them
#include <stdio.h> int main() { int check; int a, b; printf("Please input three number, separate them using space button:\n"); // Input two variables scanf("%d%d%n", &a, &b, &check); // Print value of a, b, and check printf("%d\n%d\n%d", a, b, check); return 0; }
Results:
Please input three number, separate them using space button:
10 20 5
10
20
5
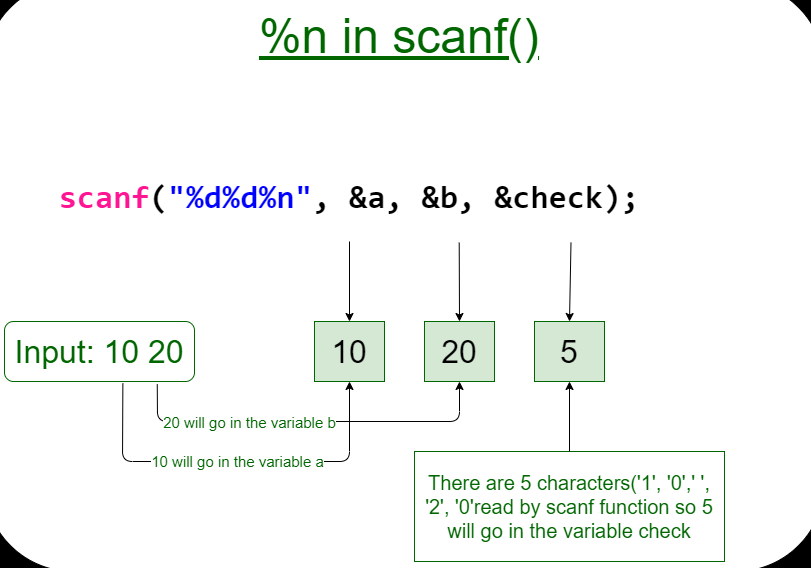
Example 2.3) Reads an Integer From the User and Prints out its Cube
#include<stdio.h> int main(){ int num; printf("enter a number:"); scanf("%d", &num); printf("cube of number is:%d ", num*num*num); return 0; }
Results:
enter a number:9
cube of number is:729
In this program, the user is prompted to enter an integer. The input is read using scanf
and stored in the variable num
. Then, the cube of the entered number is calculated by multiplying it with itself twice (num * num * num
) in printf()function. Finally, the program displays the original number and its cube using printf
.
Example 2.4) Read two Integers and Calculate The Sum
#include<stdio.h> int main(){ int x=0, y=0, result=0; printf("enter first number:"); scanf("%d", &x); printf("enter second number:"); scanf("%d", &y); result=x+y; printf("sum of 2 numbers:%d ", result); return 0; }
Results:
enter first number:5
enter second number:6
sum of 2 numbers:11
It’s crucial to be cautious when using scanf
and ensure that you include the &
operator where necessary. Beginners often overlook the &
operator, which can lead to unexpected behavior or program crashes.
In most cases, if you forget to include the &
operator before the variable name in scanf
, the program may crash or produce incorrect results. However, there are also scenarios where the program might not crash, but the input won’t be properly stored in the intended variable. These situations can be particularly challenging to debug because the compiler might not provide any warning messages.
To avoid such issues, it’s important to double-check the usage of scanf
and ensure that you correctly include the &
operator before the variables you want to read input into. Additionally, paying attention to compiler warnings and using good coding practices, such as validating user input, can help mitigate potential problems.