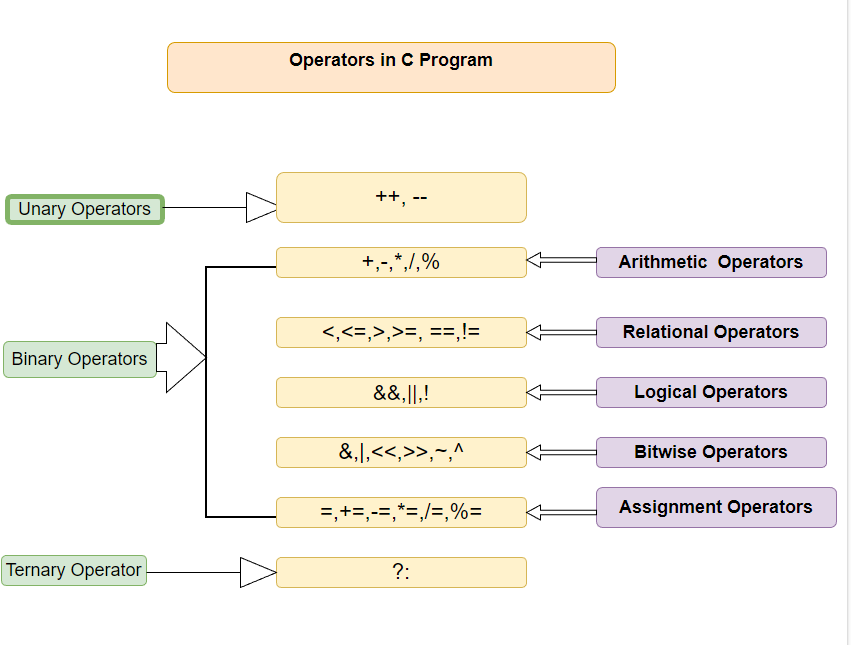
Arithmetic Operators
An arithmetic operator performs mathematical operations such as addition, subtraction, multiplication, division etc on numerical values (constants and variables).
The following table shows all the arithmetic operators supported by the C language. Assuming variable A has a value of 18 and variable B has a value of 5:
Operator | Description | Example |
---|---|---|
+ | Adds two numbers | A + B will result in 23 |
– | Subtracts one number from another | A – B will result in 13 |
* | Multiplies two numbers | A * B will result in 90 |
/ | Divides the numerator by the denominator | A / B will result in 3.6 |
% | Modulus operator, returns the remainder after division | B % A will result in 3 |
++ | Increment operator, increases the integer value by 1 | A++ will result in 19 |
— | Decrement operator, decreases the integer value by 1 | A– will result in 17 |
Example 1) Application of arithmetic operators.
#include <stdio.h> int main() { int A=18; int B=5; printf("A=%d\n", A); printf("B=%d\n",B); printf("A+B=%d\n",A+B); printf("A-B=%d\n",A-B); printf("A*B=%d\n",A*B); printf("A/B=%d\n",A/B); int C = A % B ; printf("A Remainder B=%d\n",C); A++; // to increase the value of a variable by 1 printf("A++=%d\n",A); B--; // to decrease the value of a variable by 1 printf("B--=%d\n",B); return 0; }
Results:
A=18
B=5
A+B=23
A-B=13
A*B=90
A/B=3
A Reminder B=3
A++=19
B--=4
Process returned 0 (0x0) execution time : 0.039 s
Press any key to continue.
2. C Increment and Decrement Operators
There are two ways to perform increment or decrement operations on a variable in C:
C programming has two operators increment ++
and decrement --
to change the value of an operand (constant or variable) by 1.
Increment ++
increases the value by 1 whereas decrement --
decreases the value by 1.
Post-increment:
variable++; // Represents incrementing the variable after its current value is used;
Pre-increment:
++variable; // Represents incrementing the variable before its value is used;
Post-decrement:
variable–; // Represents decrementing the variable after its current value is used;
Pre-decrement:
–variable; // Represents decrementing the variable before its value is used.
example 2 ) The increment and decrement operators
#include <stdio.h> int main() { int ii; ii=10; printf("ii=%d\n",ii); printf("ii++=%d\n",ii++); // Post-increment printf("ii=%d\n\n",ii); ii=10; printf("ii=%d\n",ii); printf("++ii=%d\n",++ii); // Pre-increment printf("ii=%d\n\n",ii); ii=10; printf("ii=%d\n",ii); printf("--ii=%d\n",--ii); // Pre-decrement printf("ii=%d\n\n",ii); ii=10; printf("ii=%d\n",ii); printf("ii--=%d\n",--ii); // Post-decrement printf("ii=%d\n\n",ii); return 0; }
results:
ii=10
ii++=10
ii=11
ii=10
++ii=11
ii=11
ii=10
--ii=9
ii=9
ii=10
ii--=9
ii=9
Process returned 0 (0x0) execution time : 0.957 s
Press any key to continue.