1. if-else-if Ladder Statements
The if-else-if ladder statement is a series of if-else statements where each ‘if’ condition is checked sequentially. If the condition of the first ‘if’ statement is true, the corresponding block of code is executed, and the rest of the conditions are skipped. If the condition is false, the program moves to the next ‘else-if’ condition, and so on. If none of the conditions is true, the code inside the ‘else’ block is executed.
Syntex
if (condition1) { // code to be executed if condition1 is true } else if (condition2) { // code to be executed if condition2 is true } else if (condition3) { // code to be executed if condition3 is true } .... // once if-else ladder can have multiple else if else { // code to be executed if none of the conditions are true }
Here’s a breakdown of the syntax:
if (condition1) { ... }
: This is the initialif
statement, wherecondition1
is the expression that is evaluated. Ifcondition1
is true, the code inside the corresponding block will be executed.else if (condition2) { ... }
: Ifcondition1
is false, thencondition2
is checked. Ifcondition2
is true, the code inside this block will be executed.else if (condition3) { ... }
: Similarly, if bothcondition1
andcondition2
are false, thencondition3
is checked. Ifcondition3
is true, the code inside this block will be executed.else { ... }
: If none of the previous conditions are true, the code inside theelse
block will be executed. This part is optional; if you don’t need to handle the case where none of the conditions are true, you can omit theelse
block.
Keep in mind that the else if
part can be repeated for as many conditions as needed, creating a ladder-like structure. The entire structure ensures that only one block of code will be executed, corresponding to the first true condition encountered from top to bottom.
A if-else-if ladder works as following diagram
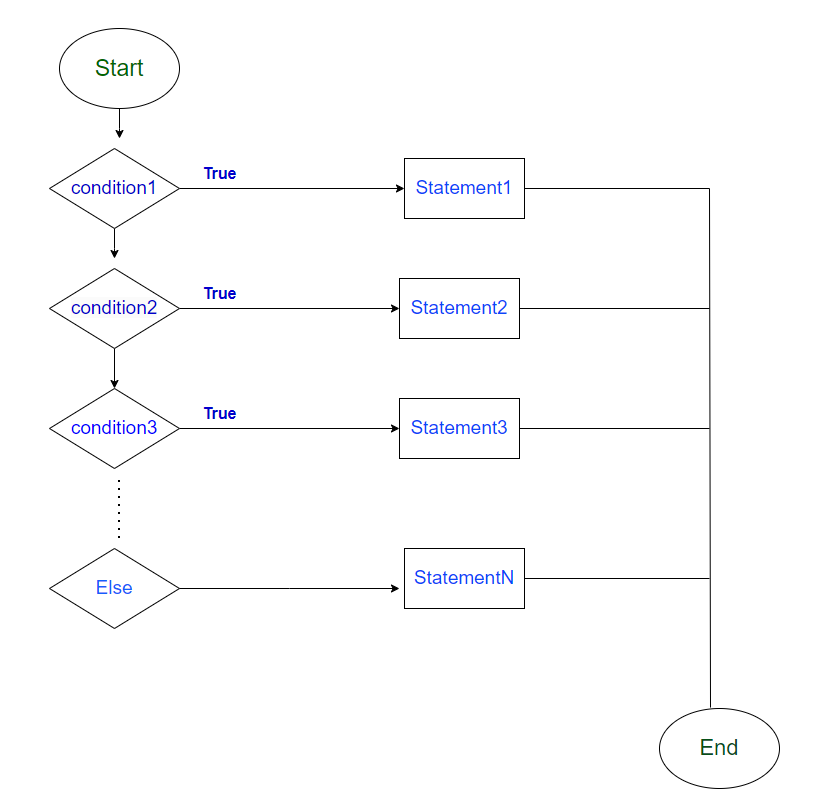
The expressions are evaluated from top to bottom. As soon as a true test expression is found, the associated statement will be executed. When all test expressions are false, the default else statement will be executed.
This structure ensures that only the statement block associated with the first true condition is executed, and the statement blocks associated with other conditions are not executed. This is an effective way to handle control flow in situations with multiple conditions.
Example 1.1 ) Print out the level of the score.
#include <stdio.h> int main() { int score = 75; const char *level; const char *result; if (score >= 90) { level = "Excellent"; result = "Outstanding"; } else if (score >= 80) { level = "Good"; result = "Above Average"; } else if (score >= 70) { level = "Average"; result = "Satisfactory"; } else if (score >= 60) { level = "Pass"; result = "Minimum Passing"; } else { level = "Fail"; result = "Failing"; } printf("Score: %d\n", score); printf("Level: %s\n", level); printf("Result: %s\n", result); return 0; }
Explanation:
- Header Inclusion:
#include <stdio.h>
includes the standard input/output library, which provides functions likeprintf
. - Function Declaration:
int main()
is the entry point of the program. It declares themain
function, which is executed when the program is run. - Variable Declaration:
int score = 75;
declares an integer variablescore
and initializes it with a value of 75. Theconst char *level
andconst char *result
declare pointers to constant characters, which will be used to store level and result strings. - Conditional Statements: The program uses a series of
if-else if
statements to determine the level and result based on the value of thescore
variable. - Print Statements:
printf
statements are used to print the score, level, and result to the console.%d
is used as a format specifier for an integer, and%s
is used for a string. - Return Statement:
return 0;
indicates that the program has executed successfully. The value 0 is returned to the operating system.
Example 1.2 ) Compare the sizes of two numbers.
// Program to compare two integers using =, > or < symbol #include <stdio.h> int main() { int number1, number2; printf("Enter two integers: "); scanf("%d %d", &number1, &number2); //checks if the two integers are equal. if(number1 == number2) { printf("Result: %d = %d",number1,number2); } //checks if number1 is greater than number2. else if (number1 > number2) { printf("Result: %d > %d", number1, number2); } //checks if both test expressions are false else { printf("Result: %d < %d",number1, number2); } return 0; }
Results:
Enter two integers: 12 23 Result: 12 < 23
2. Nested if-else Statements
It is possible to include an if...else
statement within the body of another if...else
statement.
The program provided below uses <
, >
, and =
to compare two integers, similar to the example of the if...else
ladder in Example 1.2. However, we will use nested if...else
statements to solve this problem.
Example 2.1 ) Compare the sizes of two numbers
#include <stdio.h> int main() { int number1, number2; printf("Enter two integers: "); scanf("%d %d", &number1, &number2); if (number1 >= number2) { if (number1 == number2) { printf("Result: %d = %d",number1,number2); } else { printf("Result: %d > %d", number1, number2); } } else { printf("Result: %d < %d",number1, number2); } return 0; }
If the body of an if…else statement consists of only one statement, there is no need to use curly braces {}. For example:
if (a > b) { printf("Hello"); } printf("Hi");
Equivalent to:
if (a > b) printf("Hello"); printf("Hi");
Example 2.2) Nested if else statements compare whether a number is greater than 1 or greater than 10.
#include<stdio.h> int main() { int num=1; if(num<10) { if(num==1) { printf("The value is:%d\n",num); } else { printf("The value is greater than 1"); } } else { printf("The value is greater than 10"); } return 0; }
The above program checks whether the number is greater than 10 or not and prints the results using nested if-else statements.
- First, we declare a variable
num
with a value of 1. Then, we use an if-else construct. - In the outer if-else, the provided condition checks if the number is less than 10. If the condition is true, the program enters the inner block for processing. In this example, the condition is true, so it enters the inner block for computation.
- Within the inner block, we again have a condition checking if our variable contains the value 1. When the condition is true, it processes the if block; otherwise, it processes the else block. In this case, the condition is true, so a program segment is executed, and the value is printed on the output screen.
- The above program prints the value of the variable and exits successfully.