Relational operators, also known as comparison operators, are used in C programming to establish relationships between values. Similar to mathematical expressions like 10 > 9 or 1 < x < 5, relational operators evaluate a condition and produce a result that can be either true or false. For example, the statement “1 > 2” is a false condition.
In computer programming, these relational operations are also supported, but the result is binary, meaning it can only be either true or false. When a condition is evaluated and found to be true, it is referred to as “true,” and when it is false, it is referred to as “false.” Each condition evaluation can only yield one of these two outcomes and cannot have other possibilities. Additionally, the same condition will always produce the same result, regardless of the context.
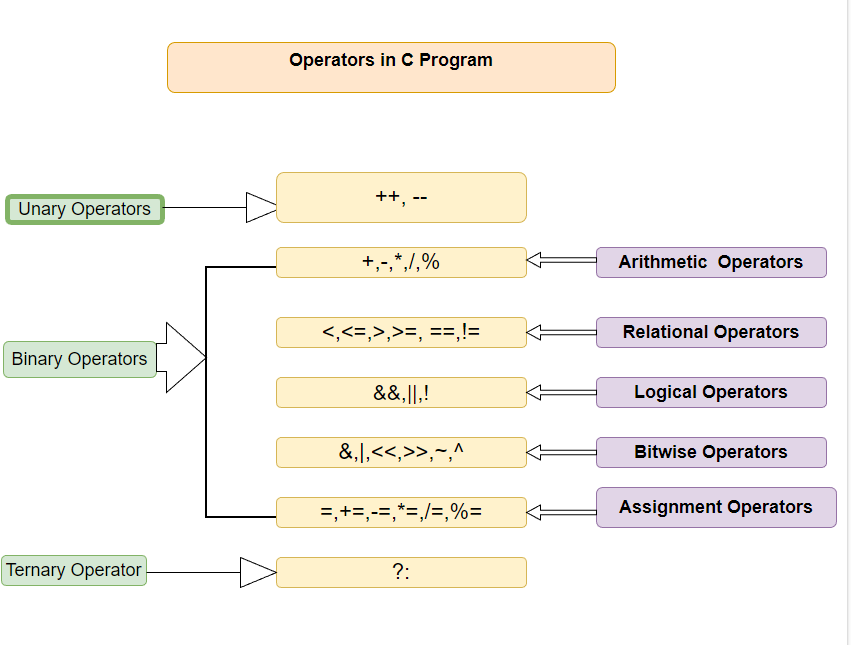
Relational operators allow us to compare values, such as checking if one value is greater than or equal to another, less than or equal to another, or equal to another. These comparisons are fundamental for decision-making and control flow in programming.
Operator | Description | Example(a and b, a = 10, b = 11) |
== |
Checks if the values of two operands are equal, and if so, the condition becomes true. | a == b, false |
!= |
Checks if the values of two operands are not equal, and if so, the condition becomes true. | a != b, true |
> |
Checks if the value of the left operand is greater than the value of the right operand, and if so, the condition becomes true | a > b, false |
< |
Checks if the value of the left operand is less than the value of the right operand, and if so, the condition becomes true | a < b, true |
>= |
Checks if the value of the left operand is greater than or equal to the value of the right operand, and if so, the condition becomes true. | a >= b, false |
<= |
Checks if the value of the left operand is less than or equal to the value of the right operand, and if so, the condition becomes true. | a <= b, true |
Example 1. Relational Operators
#include <stdio.h> int main() { int a = 10, b = 20, result; // Equal result = (a==b); printf("(a == b) = %d \n",result); // less than result = (a<b); printf("(a < b) = %d \n",result); // greater than result = (a>b); printf("(a > b) = %d \n",result); // less than equal to result = (a<=b); printf("(a <= b) = %d \n",result); return 0; }
In C programming language, true represents any value except zero. False is represented by zero.