1. break Statement
In C, the break
and continue
statements are used to control the flow of loops.
The break
statement is primarily used in the switch statement. It is also useful for immediately stopping loops.
When the break
statement is used in the do-while loop statement, for loop statement, or while loop statement, it terminates the loop and executes the statements following the loop. Typically, the break
statement is always associated with an if statement. It means that when a certain condition is met, the loop is exited.
Syntax
break;
Example 1.1 ) break statement
#include <stdio.h> int main() { int num = 5; while (num > 0) { if (num == 3) break; printf("%d\n", num); Num--;} }
Output
Num starts from five, decreasing; print 5 and 4, then directly break out of the while loop, no longer iterate. Note:
- The break statement does not affect the if-else conditional statements.
- In multi-level loops, a break statement only jumps out one level.
- When you want to exit the current loop and enter the next one, use the continue statement. This statement ignores all the statements after it in the current loop and enters the next loop. The continue statement is used only in loop bodies such as for, while, do-while, often used together with if conditional statements to speed up the loop.
Example 1.2 ) break statement in a while
loop:
#include <stdio.h> int main() { int num = 5; while (num >= 0) { printf("%d\n", num); if (num <= 4) { // Break out of the loop when num is 4 or less break; } num--; } return 0; }
In this example, the while
loop continues to execute as long as num
is greater than or equal to 0. Inside the loop, the program prints the current value of num
. The if
statement checks if num
is less than or equal to 4, and if true, the break
statement is executed, causing the program to exit the loop immediately.
The break
statement is particularly useful when you want to terminate a loop based on a certain condition, without necessarily completing all iterations of the loop. It provides a way to jump out of the loop and continue with the rest of the program.
Here’s a step-by-step explanation of how the program works:
- The
num
variable is initialized to 5. - The
while
loop is entered, and the conditionnum >= 0
is true, so the loop executes. - Inside the loop, it prints the current value of
num
, which is 5, and then decrementsnum
by 1 (num--
). - The
if
statement checks ifnum
(now 4) is less than or equal to 4, which is true. - The
break
statement is executed, causing the program to exit thewhile
loop immediately. - The program continues with the next statement after the loop, which is the
return 0;
statement, and the program terminates.
Output:
5 4
2. continue Statement
In C, the continue
statement is used to skip the rest of the code inside a loop for the current iteration and jump to the next iteration of the loop. It is often used in conjunction with an if
statement to conditionally decide whether to skip the remaining code in the loop for a particular iteration.
Syntax:
continue;
The continue
statement is almost always used with the if...else
statement.
Example 2.1 ) continue Statement in C Loop
#include <stdio.h> int main() { for (int i = 1; i <= 5; i++) { // Skip the loop iteration if i is even if (i % 2 == 0) { continue; } // Print the value of i for odd values only printf("%d\n", i); } return 0; }
Output
1 3 5
Explanation:
- The
for
loop initializesi
to 1 and iterates as long asi
is less than or equal to 5. - Inside the loop, there’s an
if
statement checking ifi
is even (i % 2 == 0
). - For the first iteration (when
i = 1
), the condition is false, and theprintf
statement is executed, printing1
. - For the second iteration (when
i = 2
), the condition is true, and thecontinue
statement is executed. This skips theprintf
statement and jumps to the next iteration of the loop. - The pattern continues for each iteration: the
printf
statement is executed for odd values ofi
and skipped for even values. - The final output includes only the values of
i
that are odd (1, 3, and 5).
In summary, the continue
statement allows you to skip the remaining code inside the loop for a particular iteration, providing a way to control the flow of the loop based on specific conditions.
Example 2.2) Print numbers within 7 excluding 5.
#include <stdio.h> int main() { int nb = 7; while (nb > 0) { nb--; if (nb == 5) continue; printf("%d\n", nb); } }
Output
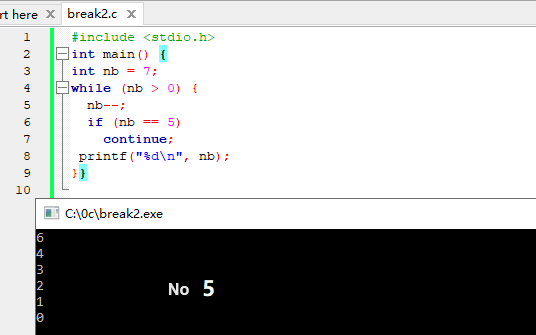
Example 2.3) Print the characters entered by the user, press Enter to exit, and do nothing if the ESC key is pressed.
main() { char c; while(c!=13) { c=getch(); if(c==0X1B) continue; printf("%c\n", c); } }
Output:
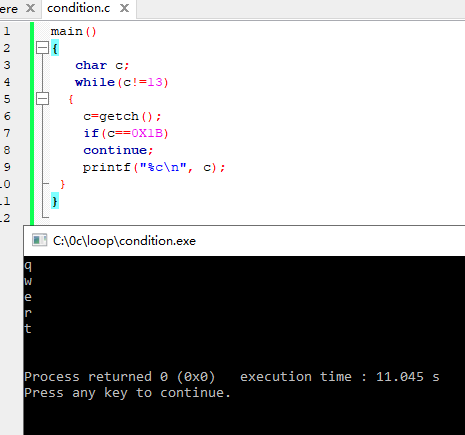
ASCII code: 13 represents the Enter key, 0x1B represents ESC (escape), and the Overflow key.