printf()
and scanf()
are both functions in the C programming language that deal with input and output, but they serve different purposes.
printf()
is used for formatted output, which means it allows you to display formatted text and variables on the screen or send them to a file. It takes a format string as an argument that specifies the desired format and uses placeholders (format specifiers) to represent the variables’ values. It is primarily used for displaying information to the user or generating formatted output.
On the other hand, scanf()
is used for formatted input, which means it allows you to read and parse input from the user or a file. It also takes a format string as an argument, which specifies the expected format of the input. It uses placeholders (format specifiers) to indicate the data types and locations where the input values should be stored. It is commonly used for accepting user input during runtime.
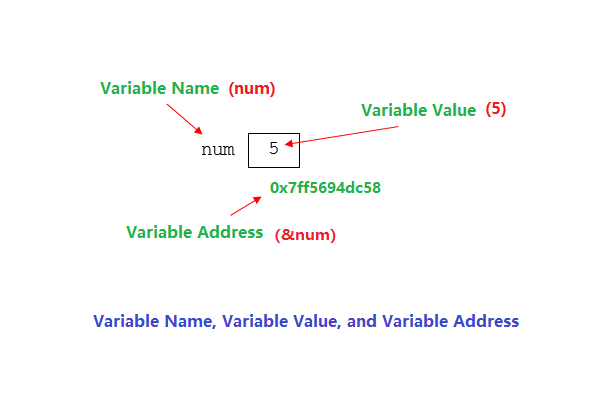
The Difference Between printf() and scanf()
1. float and double types
The length modifiers and conversion specifiers in scanf()
and printf()
are almost the same, but there is a key difference for float and double types.
In printf()
, the conversion specifier for both float and double types is f
. However, in scanf()
, the float type remains the same, but the double type requires a length modifier l
before the conversion specifier f
, resulting in %lf
.
#include<stdio.h> int main(void) { double a = 3.0; double b; scanf("%lf", &b); printf("%lf\n", a); printf("%lf", b); return 0; }
Results:
15 3.000000 15.000000
The scanf() function follows certain rules to recognize integers or floating-point numbers.
When reading integers, the scanf() function first looks for a plus sign (+) or minus sign (-), and then it starts reading digits until it encounters a non-digit character.
When reading floating-point numbers, the scanf() function first looks for a plus sign (+) or minus sign (-) (optional), followed by a sequence of digits (which may include a decimal point), and then an exponent (optional). The exponent consists of the letter “e”, an optional sign, and one or more digits.
If scanf() encounters a character that cannot be part of the current item being read, it will “put back” that character in its place, so that it can be read again during the next item scanning or the next call to scanf().
These rules help scanf() recognize and interpret the input correctly based on the specified format in the format string.
2. Confusion Between scanf() and printf()
printf("%d", &i);
The output is not the value of i
but the decimal value of the address of i
.
scanf("%d, %d", &i, &j);
After scanf()
reads an integer using the first %d
, it attempts to match the comma (,
) with the next character in the input stream. If this character is not a comma, scanf()
will terminate without reading the value for the variable j
.
scanf("%d\n", &i);
In the printf()
function, it is common to use \n
to insert a newline character, which causes a line break. However, if you place a \n
at the end of the format string in scanf()
, it can lead to unexpected behavior.
In scanf()
, the newline character \n
is treated as whitespace. Therefore, scanf()
will search for whitespace characters in the input stream. As mentioned earlier, whitespace characters in the scanf()
format string match zero or more whitespace characters in the input stream. So when you enter input and press the Enter key, the newline character will match the \n
in scanf()
, and subsequent presses of the Enter key alone will not cause scanf()
to terminate unless you input a non-whitespace character that causes scanf()
to fail and exit.
It’s important to be aware of this behavior to ensure proper input handling in your scanf()
statements and avoid unexpected issues when using \n
in the format string.