In the C language, statements are primarily classified into three types: sequential statements, branch statements, and loop statements. In C programming, the terms “sequential statements,” “branch statements,” and “loop statements” refer to different types of statements used to control the flow of a program.
A Japanese computer engineer metaphorically likened the execution flow of a program to the flow of a river. In the process of program execution:
- The process, akin to water flowing in a single direction, is referred to as the ‘sequential execution structure.’
- The process, resembling water encountering a large rock or a watershed in the middle of the river, resulting in streams flowing in different directions, is termed as the ‘selection structure’ (conditional branching structure).
- The process, similar to water encountering obstacles and forming whirlpools, is called the ‘loop structure.
1. Sequential Statements
Sequential statements are executed in a top-down order, one after the other. Sequential structure is the fundamental structure of the C language. The program runs from top to bottom, executing one statement after another. Sequential structure is commonly used in everyday programming. It encompasses basic arithmetic operations such as addition (+), subtraction (-), multiplication (*), division (/), and also includes the frequently used modulus operator (%) for finding remainders.
The program flow moves from one statement to the next in a sequential manner. Each statement is executed once the preceding statement has been completed.
The Diagram of Sequential Statement is as Following figure.
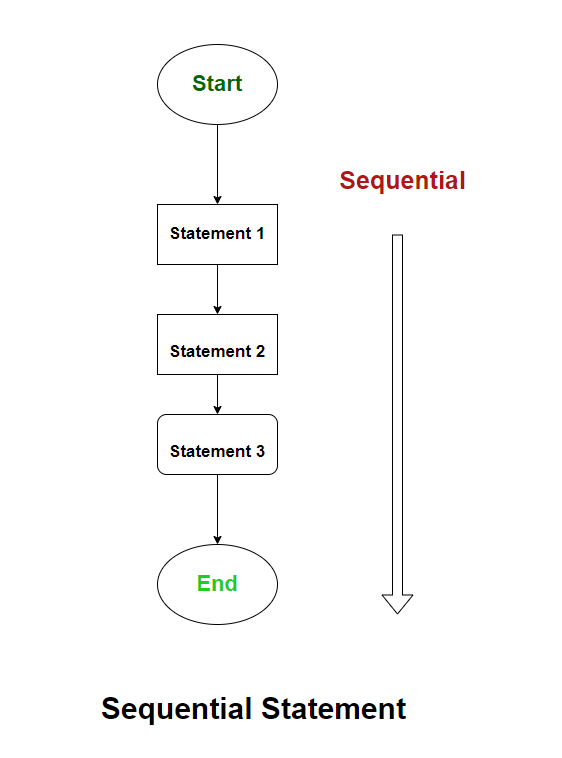
Here’s a simple example of sequential statements in C:
Example 1.1 a simple example of sequential statements
#include <stdio.h> int main() { // Sequential statements int x = 5; float y = 3.14; printf("The value of x is %d\n", x); printf("The value of y is %f\n", y); return 0; }
In this example, the program starts by declaring and initializing variables x
and y
. The printf
statements then display the values of these variables. The flow of execution follows a sequential order, moving from the declaration to the print statements. Sequential statements are the foundation of the program’s control flow, and they represent the basic structure for the execution of code in most programming languages, including C.
Example 1.2 The chicken-rabbit cohabitation problem*
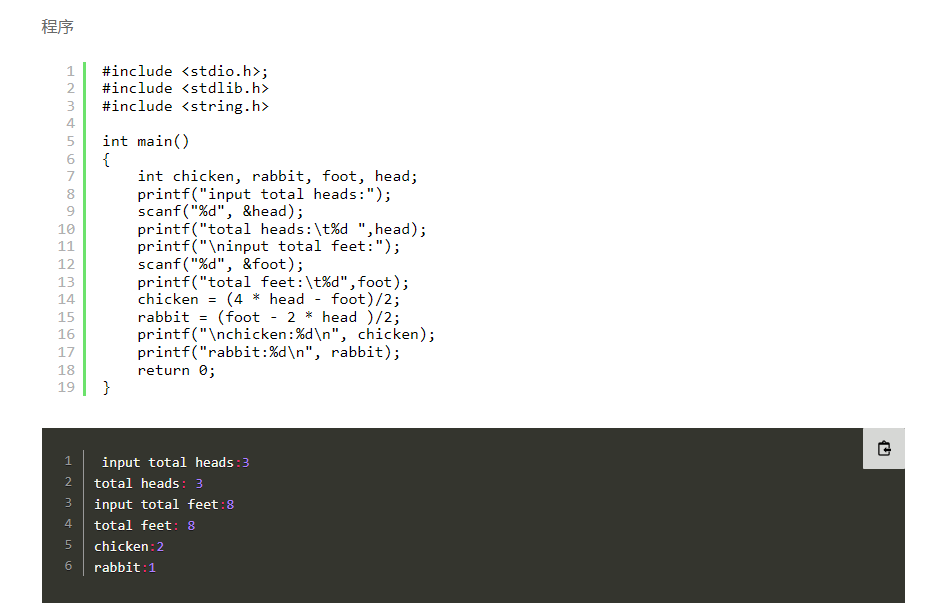
1.1* What is The Chicken-rabbit Cohabitation Problem
First, let’s understand the primary school problem of ‘chicken and rabbit in the same cage.’
Given the total number of heads (h) and the total number of legs (f), where the number of chicken heads is represented by ‘chicken’ and the number of rabbit heads is represented by ‘rabbit.’
The equations governing the problem are:
- chicken + rabbit = h;
- 2 * chicken + 4 * rabbit = f;
Solving this system of simultaneous equations, we obtain:
The number of chicken heads = (4 * total heads – total legs) / (4 – 2)
The number of rabbit heads = (total legs – 2 * rabbit heads) / (4 – 2)
2. Branch Statements
Branch statements in C involve conditional execution of code based on certain conditions. They allow the program to make decisions and choose different paths of execution depending on whether a specified condition is true or false. The primary branch statements in C are the if
, else if
, else
, and switch
statements.
The Diagram is as following:
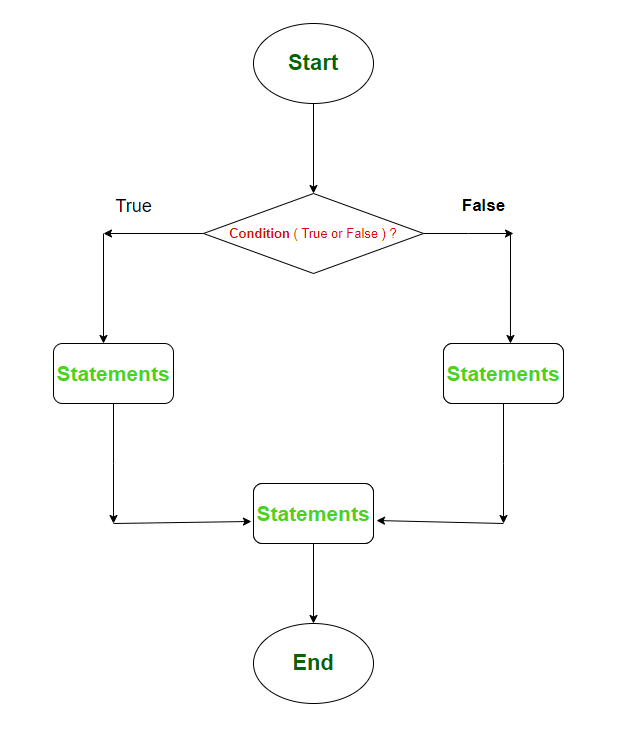
2.1 if Statement
The if
statement is used to conditionally execute a block of code if a specified condition evaluates to true.
Syntex:
if (expression) statement else statement
example 2.1 if statement
int x = 10; if (x > 0) { printf("x is positive\n"); }
2.2 else -if Statement
The if-else-if
statement allows you to test multiple conditions. If the first if
condition is false, it checks the next condition.
Syntex:
if (expression) statement else if (expression) statement ... else if (expression) statement else statement
Example 2.2.1 Example
int x = 0; if (x > 0) { printf("x is positive\n"); } else if (x < 0) { printf("x is negative\n"); } else { printf("x is zero\n"); }
2.3 switch Statement
The switch
statement is used to select one of many code blocks to be executed. It is often used when there are multiple cases to consider. The switch
statement in C is used for making decisions based on the value of an expression. It provides an alternative to using a series of if
and else if
statements when you need to compare a variable against multiple values.
Syntex:
switch (expression) { case value1: statement case value2: statement default: statement }
Example 2.3.1 switch
statement in C
#include <stdio.h> int main() { int day = 3; switch (day) { case 1: printf("Monday\n"); break; case 2: printf("Tuesday\n"); break; case 3: printf("Wednesday\n"); break; case 4: printf("Thursday\n"); break; case 5: printf("Friday\n"); break; case 6: printf("Saturday\n"); break; case 7: printf("Sunday\n"); break; default: printf("Invalid day\n"); } return 0; }
In this example, the switch
statement checks the value of the variable day
. Depending on the value of day
, the corresponding case
block is executed. If day
does not match any of the specified cases, the default
block is executed.
Results for day = 3
:
Wednesday
The break
statement is used to exit the switch
statement once a match is found. If a break
statement is not used, execution will “fall through” to the next case.
In this example, the value of day
is 3
, so the program executes the code in the case 3
block and prints “Wednesday.” The switch
statement provides a concise way to handle multiple cases based on the value of an expression.
3. Loop Statements
Loop statements in C are used to repeatedly execute a block of code as long as a certain condition is true. The Diagram of loop statements is shown as following figure:
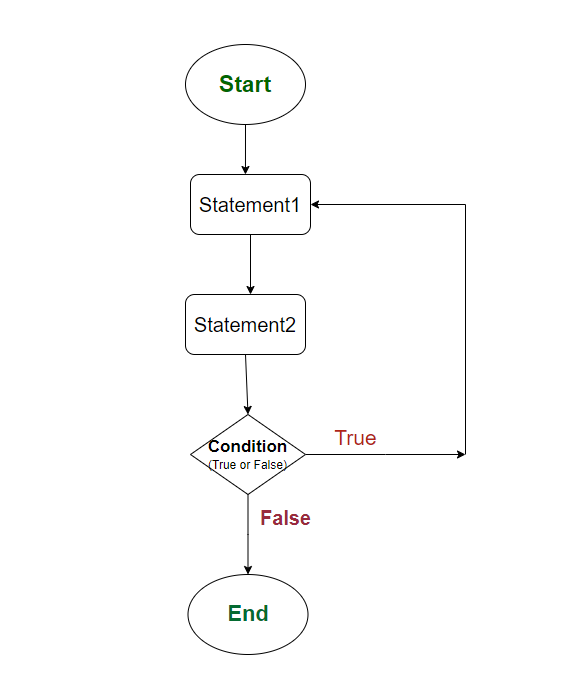
There are different types of loop statements in C, including the for
loop, while
loop, and do-while
loop.
Here’s an example using the for
loop:
#include <stdio.h> int main() { // Example of a for loop for (int i = 1; i <= 5; i++) { printf("Iteration %d\n", i); } return 0; }
In this example, the for
loop initializes a loop control variable i
to 1
, executes the loop body as long as i
is less than or equal to 5
, and increments i
by 1
after each iteration. The loop body simply prints the current iteration number.
Results:
Iteration 1
Iteration 2
Iteration 3
Iteration 4
Iteration 5
The loop continues to execute until the condition i <= 5
becomes false.
Other types of loops include the while
loop and the do-while
loop:
#include <stdio.h> int main() { // Example of a while loop int j = 1; while (j <= 5) { printf("Iteration %d\n", j); j++; } // Example of a do-while loop int k = 1; do { printf("Iteration %d\n", k); k++; } while (k <= 5); return 0; }
Both the while
loop and do-while
loop have similar functionality, but the key difference is that the do-while
loop always executes the loop body at least once, even if the condition is initially false.