1. Branch statements in C programming
Branch statements in C programming are used to make decisions based on conditions. They allow the program to execute different blocks of code depending on whether a given condition is true or false. The primary branch statements in C are the if
, else if
, else
, and switch
statements.
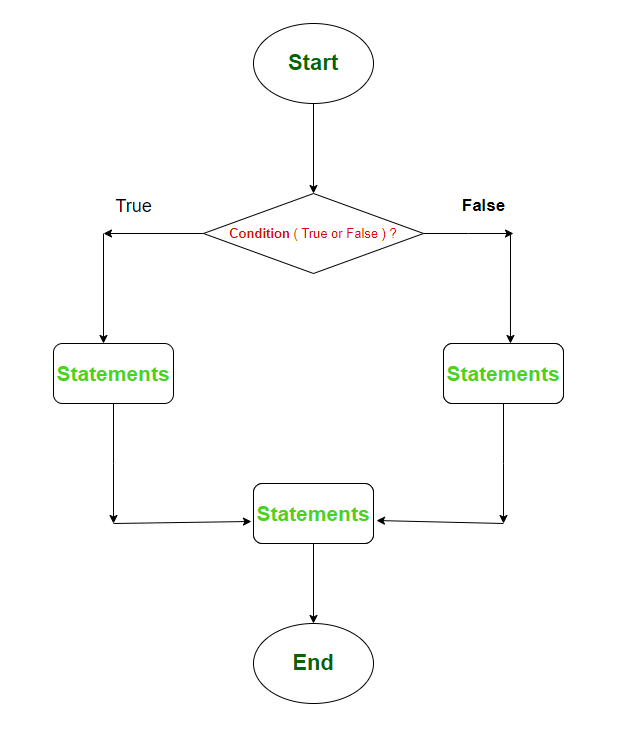
Branching Statements in C are those statements in C language that helps a programmer to control the flow of execution of the program according to the requirements. These Branching Statements are considered an essential aspect of the Programming Languages and are essentially used for executing the specific segments of code on basis of some conditions.
1.1 Types of Branch Statements in C Programming
The Branching Statements in C are categorized as follows.
- Conditional Branching Statements in C
- if Statement
- if-else Statement
- if-else-if Ladder Statement
- switch Statement
- Unconditional Branching Statements in C
- goto Statement
- break Statement
- continue Statement
2. if Statement
The if
statement is used to conditionally execute a block of code if a specified condition evaluates to true.
Syntex
if (condition) { // Statements if true }
Here in the above syntax “condition” is a logical expression that is evaluated for being true or false. If the condition is evaluated to be true, the code within curly braces will be executed but if the condition is false, the code in braces will be skipped.
2.1 Example
#include <stdio.h> int main() { int x = 10; if (x > 0) { printf("x is positive\n"); } return 0; }
Results:
x is positive
2.2 Example : Determining if an integer is 10
#include <stdio.h>; #include <stdlib.h> #include <string.h> int main() { int x; if (x == 10); printf("x is 10"); return 0; }
results:
x is 10
If there are multiple statements, they need to be placed inside curly braces to form a compound statement.
2.3 Example: Checking whether an integer is 4, and if it is not, print a sentence
#include <stdio.h>; #include <stdlib.h> #include <string.h> int main() { int x; printf("please input a number that smaller than 10\n"); scanf ("%d",&x); if (x != 4);{ printf("x is %d\n",x); printf("please input 4 next time"); } return 0; }
Ask the user to input a number that is less than 10, If it is not 4, it will print “x is XXX” Otherwise, it will print “please input 4 next time” XXX-is what the user input.
Results:
please input a number that smaller than 10 6 x is 6 please input 4 next time
3. if-else Statement
The else Statement in C programming is considered just the opposite of the if statement. This statement is used to execute the code if the condition specified in the if statement evaluates to false.
Syntex:
if (condition) { // statemnets if condition is true } else{ // statemnets if condition is false }
3.1 Example
#include <stdio.h> int main() { int x = 20; if (x < 10) { printf("x is less than 10\n"); } else { printf("x is greater than or equal to 10\n"); } return 0; }
results:
x is greater than or equal to 10
3.2 Example : checking if a letter is a vowel or a consonant.
4. if-else-if Ladder Statement
The else if
statement allows you to test multiple conditions. If the first if
condition is false, it checks the next condition.
Syntex:
if (condition1) { // code to be executed if condition1 is true } else if (condition2) { // code to be executed if condition2 is true and condition1 is false }
The “else if” statement can be repeated multiple times to check for multiple conditions. If all conditions are false, the code within the final “else” block will be executed.
4.1 Example
#include <stdio.h> int main() { int x = 0; if (x > 0) { printf("x is positive\n"); } else if (x < 0) { printf("x is negative\n"); } else { printf("x is zero\n"); } return 0; }
Results:
x is zero
In the above code, we have used a complete block of if, else if and else statements. The if statement first checks whether the value of x is greater than 0 or not. Since x (which is 0) is equal to 0, so the control moves to the else if block. Now the program checks whether x is less than 0 or not. This time the condition is false, so the code block inside this else-if block will not be executed and go to next else block and output on the screen “x is zero”.
5. switch Statement
The switch
statement is used to select one of many code blocks to be executed based on the value of an expression. It is often used as an alternative to multiple “if” and “else if” statements.
Syntax
switch (expression) { case value1: // code to be executed if expression equals value1 break; case value2: // code to be executed if expression equals value2 break; ... default: // code to be executed if none of the cases match break; }
The “expression” in the above syntax is the value being evaluated. Each “case” statement represents a possible value of the expression. If the expression matches one of the “case” statements, the code block within the matching “case” statement is executed. If none of the “case” statements match, the code within the “default” block is executed.
5.1 Example
#include <stdio.h> int main() { int day = 3; switch (day) { case 1: printf("Monday\n"); break; case 2: printf("Tuesday\n"); break; case 3: printf("Wednesday\n"); break; // ... other cases ... default: printf("Invalid day\n"); } return 0; }
Results:
Wednesday
6. Short-Circuit Evaluation
The &&
(logical AND) and ||
(logical OR) are logical operators in the C language, and they follow the principle of short-circuit evaluation. This means that when evaluating these operators, if the value of the left operand is sufficient to determine the overall result of the expression, the right operand will not be evaluated.
Short-Circuit AND (&&
): If the left operand is false, the entire expression will be false, and the right operand will not be evaluated, as the overall result is already determined to be false.
if (x > 0 && y > 0) { // Execute this code only if both x and y are greater than 0 }
Short-Circuit OR (||
): If the left operand is true, the entire expression will be true, and the right operand will not be evaluated, as the overall result is already determined to be true.
if (x == 0 || y == 0) { // Execute this code if either x or y is equal to 0 }
This short-circuit behavior is useful in certain situations, helping to improve program performance and prevent unnecessary computations.
6.1 Example short-circuit evaluation
#include <stdio.h>; #include <stdlib.h> #include <string.h> int main() { int i = 0, j = 1; if (i && i++) { ; // 空语句 } printf("%d\n", i); // The value of i has not increased, indicating that i++ has not been computed. if (j || j++) { ; } printf("%d\n", j); // The value of j has not increased, indicating that j++ has not been computed. return 0; }
Results:
0 1