In C programming, a for
loop is a control flow statement that allows you to repeatedly execute a block of code a certain number of times. The general syntax of a for
loop is as follows:
Syntax:
for (initialization; condition; updationStatement) { Statements // code to be executed }
Here’s a breakdown of the components:
- Initialization: It is an expression that is executed only once at the beginning of the loop. It typically initializes a loop control variable.
- Condition: It is a boolean expression that is evaluated before each iteration. If the condition is true, the loop body is executed; if false, the loop terminates.
- updationStatement: normally is Increment/Decrement. It is an expression that is executed after each iteration. It typically increments or decrements the loop control variable.
- Loop Body: It is the block of code enclosed in curly braces
{}
. This code is executed as long as the condition remains true.
The Diagram of for loop is as following diagram:
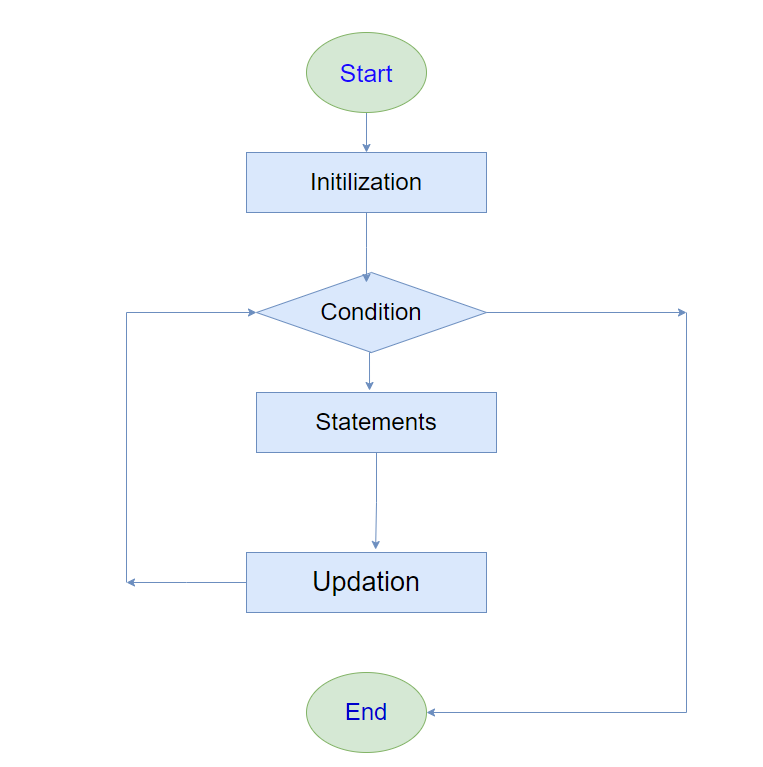
Example 1.1) prints numbers from 1 to 5
#include <stdio.h> int main() { for (int i = 1; i <= 10; i++) { printf("%d ", i); } return 0; }
In this example:
- The loop control variable
i
is initialized to 1. - The condition checks if
i
is less than or equal to 5. - The loop body prints the value of
i
. - After each iteration,
i
is incremented by 1.
The output will be: 1 2 3 4 5
Example 1.2 ) Calculating the sum of first n natural numbers
// Program to calculate the sum of first n natural numbers // Positive integers 1,2,3...n are known as natural numbers #include <stdio.h> int main() { int num, count, sum = 0; printf("Enter a positive integer: "); scanf("%d", &num); // for loop terminates when num is less than count for(count = 1; count <= num; ++count) { sum += count; } printf("Sum = %d", sum); return 0; }
Result
Enter a positive integer: 10 Sum = 55
2.Ten key Points to Note for the for
Loop Statement
1.In the for
loop, loop variable initialization, loop condition, and loop variable updation are optional, meaning they can be omitted, but the “;” cannot be omitted.
2. If loop variable initialization)” is omitted, it implies not assigning an initial value to the loop control variable.
3. If loop condition is omitted, it results in an infinite loop when no other action is taken.
for(i=1;;i++) sum=sum+i;
Equivalent to:
i=1; while(1) { sum=sum+i; i++; }
4. Omitting variable updation means no operation is performed on the loop control variable. In this case, you can include statements within the loop body to modify the loop control variable.
For example:
for(i=1;i<=100;){ sum=sum+i; i++; }
5. Omitting initialization and loop variable updation.
For example:
for(;i<=100;){ sum=sum+i; i++; }
Equivalent to:
while(i<=100){ sum=sum+i; i++; }
6. for (;;)
equivalent to: while(1)
7. Initilization statement and updation statement can be a simple expression or a comma expression.
for(sum=0,i=1;i<=100;i++)sum=sum+i;
and
for(i=0,j=100;i<=100;i++,j--)k=i+j;
both are OK.
8. Condition Statement : The condition in the loop is generally a relational expression or a logical expression, but it can also be a numeric expression or a character expression. As long as its value is non-zero, the loop body is executed.
for(i=0;(c=getchar())!=’\n’;i+=c);
and
for(;(c=getchar())!=’\n’;) printf(“%c”,c);
9. In a for
loop, each part can have multiple expressions separated by commas.
for (x = 0, y = num; x < y; i++, y--) { statements; }
10. We can place the initialization expression outside the for
loop by adding a semicolon to skip the initialization expression. For example:
int i=0; int max = 10; for (; i < max; i++) { printf("%d\n", i); }
3. for Loop Statement and Loop Nesting in
Loop nesting refers to the practice of placing one or more loops inside another loop. This is done to create more complex looping patterns and to solve problems that require multiple levels of iteration.
Example 3.1) Loop nesting with an outer loop running
#include <stdio.h> int main() { // Example of a simple for loop printf("Example of a simple for loop:\n"); for (int i = 1; i <= 5; i++) { printf("%d ", i); } printf("\n"); // Example of loop nesting printf("\nExample of loop nesting:\n"); for (int outer = 1; outer <= 3; outer++) { printf("Outer Loop Iteration %d:\n", outer); for (int inner = 1; inner <= 2; inner++) { printf(" Inner Loop Iteration %d\n", inner); } } return 0; }
- The first part of the code demonstrates a simple
for
loop that prints numbers from 1 to 5. - The second part illustrates loop nesting, where an outer loop runs three times, and for each iteration of the outer loop, an inner loop runs twice. This results in a total of 3 (outer) * 2 (inner) = 6 iterations.
Output
Example of a simple for loop: 1 2 3 4 5 Example of loop nesting: Outer Loop Iteration 1: Inner Loop Iteration 1 Inner Loop Iteration 2 Outer Loop Iteration 2: Inner Loop Iteration 1 Inner Loop Iteration 2 Outer Loop Iteration 3: Inner Loop Iteration 1 Inner Loop Iteration 2
This output demonstrates the execution of both a simple for
loop and loop nesting in a C program.
Nesting loops can occur an infinite number of times. When programming, remember to align each loop properly, making it easy to read and understand.
Do it for yourself and for others. Sometimes, when you revisit a program you wrote after some time, it might not be very clear.
Nesting loops are often used in array and matrix operations.
Example 3.2) The nested for
loop statement outputs a multiplication table.
#include <stdio.h> int main() { int i, j; int table = 2; int max = 5; for (i = 1; i <= table; i++) { // outer loop for (j = 0; j <= max; j++) { // inner loop printf("%d x %d = %d\n", i, j, i*j); } printf("\n"); /* blank line between tables */ } }
Output
4.Summary
- Initialization: The initialization expression is executed only once at the beginning of the loop. It typically initializes a loop control variable.
- Condition: The loop continues as long as the condition is true. If the condition is false at the beginning, the loop body is not executed.
- Updation Statement (Increment/Decrement): The increment/decrement expression is executed after each iteration of the loop. It typically modifies the loop control variable.
- Loop Control Variable: The loop control variable is often used to control the number of iterations.
- Entry-controlled: The
for
loop is an entry-controlled loop, as the condition is checked before entering the loop body. - Pre-check Loop: The condition is evaluated before the execution of the loop body. If the condition is false initially, the loop body is not executed at all.
- Termination: The loop terminates when the condition becomes false.
- Use Cases: The
for
loop is suitable when the number of iterations is known beforehand and can be controlled by a loop control variable.