The switch
statement in the C language is a structure used for multi-branch selection. It is commonly used to execute different code blocks based on the value of an expression.
1. switch Statements
In the C language, the switch statement tests the value of a variable in the expression and compares it sequentially with various cases. Once a match is found, the statement block specified for that match is executed.
The switch statement allows us to execute a code block among many alternatives.
You can achieve the same with an if…else if ladder. However, the syntax of the switch statement is more readable and writable.
A switch statement allows testing a variable for equality against multiple values. Each value is called a case, and the tested variable is checked against each switch case.
If no value matching the expression variable is found, the default statement is executed, and control will exit the switch statement.
Syntax
switch (expression) { case constant1: // Code block 1 for constant1 break; case constant2: // Code block 2 for constant2 break; // More case statements can be added as needed default: // Default code block, optional }
- In the switch statement, the
expression
is a constant expression and must be of integer or character type. - You can have any number of
case
statements within a switch. Eachcase
is followed by a value to compare (constant1, constant2, … constant-n) and a colon. - The constant values (constant1, constant2, … constant-n) in each
case
must have the same data type as theexpression
variable in the switch and must be constants. - When the tested variable is equal to the constant in a
case
, the statements following thatcase
(Block-1, or Block-2, or Block-n) will be executed until abreak
statement is encountered. - When a
break
statement is encountered, the switch terminates, and control flow jumps to the next line after the switch statement (Statement-x). - Each
case
should include abreak
(recommended, but if not included, the program becomes more complex). - A switch statement can have an optional
default
case, which appears at the end of the switch. Thedefault
case is executed when none of the preceding cases is true. - A
break
statement is not required in thedefault
case.
The following diagram illustrates the selection of each case and how the control flow exits.
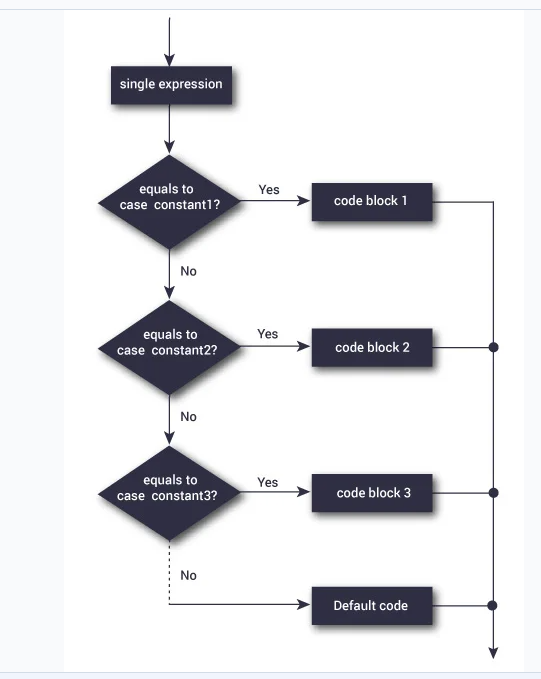
Example 1.1 ) A simple calculator
A simple calculator can be implemented using a switch
statement. Users can input two operands and an operator, and the program calculates and outputs the result. Here’s a simple example program:
// Program to create a simple calculator #include <stdio.h> int main() { char operation; double n1, n2; printf("Enter an operator (+, -, *, /): "); scanf("%c", &operation); printf("Enter two operands: "); scanf("%lf %lf",&n1, &n2); switch(operation) { case '+': printf("%.1lf + %.1lf = %.1lf",n1, n2, n1+n2); break; case '-': printf("%.1lf - %.1lf = %.1lf",n1, n2, n1-n2); break; case '*': printf("%.1lf * %.1lf = %.1lf",n1, n2, n1*n2); break; case '/': printf("%.1lf / %.1lf = %.1lf",n1, n2, n1/n2); break; // operator doesn't match any case constant +, -, *, / default: printf("Error! operator is not correct"); } return 0; }
This simple calculator program prompts users to input two operands and an operator, then uses a switch
statement to perform the corresponding calculation based on the operator and outputs the result. It checks for division by zero during division operations to avoid errors.
Result:
Enter an operator (+, -, *, /): - Enter two operands: 32.5 12.4 32.5 - 12.4 = 20.1
The user-input subtraction operator is stored in the operator
variable. Additionally, two operands, 32.5 and 12.4, are respectively stored in the variables n1
and n2
.
Due to the subtraction operation, the program’s control jumps to the corresponding code block.
printf("%.1lf - %.1lf = %.1lf", n1, n2, n1-n2);
Finally, the break
statement terminates the switch
statement.
Example 1.2 ) Check if an integer is 8.
#include <stdio.h> int main() { int num = 8; switch (num) { case 7: printf("Value is 7"); break; case 8: printf("Value is 8"); break; case 9: printf("Value is 9"); break; default: printf("Out of range"); break; } return 0; }
Results:
Value is 8
The Explanation of above example
- Assign the initial value of 8 to the integer variable
num
. - The switch statement begins comparing the values of each case with the value stored in the variable
num
. - In this program, since the value stored in the variable
num
is 8, when it reaches case 8,num
finds a matching value, executes the print statement, and then breaks out of the switch.
Example 1.3) Represent an integer from 1 to 7 in words
main(){ int a; printf("input integer number between 1 - 7: "); scanf("%d",&a); switch (a) { case 1: printf("Monday\n"); break; case 2: printf("Tuesday\n"); break; case 3: printf("Wednesday\n"); break; case 4: printf("Thursday\n"); break; case 5: printf("Friday\n"); break; case 6: printf("Saturday\n"); break; case 7: printf("Sunday\n"); break; default: printf("error\n"); break; } }
If “break” is not used, the program will execute all subsequent statements.
Example 1.4) Another usage of the switch statement
#include <stdio.h> int main() { int number=5; switch (number) { case 1: case 2: case 3: printf("One, Two, or Three.\n"); break; case 4: case 5: case 6: printf("Four, Five, or Six.\n"); break; default: printf("Greater than Six.\n"); } }
Results:
The switch statement has another usage, which involves omitting the break statement within cases to share a common code block among multiple cases. This way, when a particular case is matched, the program continues to execute subsequent case statements without exiting the switch statement.
In this example, if the value of number is 4, 5, or 6, the program will execute the code block after the sixth case without exiting the switch. If number doesn’t match any case, the code block after the default will be executed.
This usage helps reduce code redundancy, but it’s important to ensure the correctness of the program logic when using it.
2.Embeded switch Statement
As the name suggests, a nested switch statement is a switch statement inside another switch statement.
Example 2.1 ) Check username and password
#include <stdio.h> int main() { int ID = 500; int password = 000; printf("Plese Enter Your ID:\n "); scanf("%d", & ID); switch (ID) { case 500: printf("Enter your password:\n "); scanf("%d", & password); switch (password) { case 000: printf("Welcome Dear Programmer\n"); break; default: printf("incorrect password"); break; } break; default: printf("incorrect ID"); break; } }
The scanf
command stores characters or integers from standard input into the addresses of variables.
- Two initialized integer variables: ID and password.
- An external switch statement compares the input value of ID, executes the statement block that matches its value (when ID == 500).
- In the case of a correct ID, an internal switch statement is used to compare the input value of the password and execute the statement block linked to the matched value (when password == 000). If the password is incorrect, it will print “Incorrect password.”
- If the ID input is incorrect, the program will print “Incorrect ID” without entering the internal switch statement.
Why we Need switch Statement ?
The switch
statement is a control flow structure used to handle multiple possible cases. The if-else statement poses a potential issue, namely, as the number of alternative paths increases, the complexity of the program also increases. If multiple if-else constructs are used in the program, it may become challenging to read and understand.
switch statement provides a clear and efficient way to choose different execution paths based on the value of an expression. Here are some reasons for using the switch
statement:
- Multiple Options: When there are multiple possible cases to handle, using
switch
can be clearer and more readable than a series of nestedif-else
statements. - Structural Clarity: The
switch
statement offers a structurally clear way to organize code, making it easier to understand and maintain. - Performance: In certain situations,
switch
may be more efficient than an equivalent series ofif-else
statements because it often uses a jump table, which can make case matching faster. - Readability: For specific cases where choices are based on discrete values like integers or characters, a
switch
statement can be more natural and easier to comprehend. - Avoiding Code Duplication: By consolidating the execution code blocks for multiple cases,
switch
can help avoid repeating the same code for different cases.
While the switch
statement is useful in certain scenarios, it may not always be the optimal choice in other situations. In practical programming, the decision to use switch
or other control structures often depends on specific requirements and code readability.
Summary
- The switch statement is a decision-making statement in the C language.
- It is used in programs involving multiple decisions.
- A switch must include an executable expression.
- Each case must contain a break.
- Case values must be constants and unique.
- The default case is optional.
- Multiple switch statements can be nested within each other.