A loop statement in programming is a construct that allows a set of instructions to be repeatedly executed based on a certain condition. It enables the automation of repetitive tasks, making the code more concise and efficient. The loop statement keeps iterating over a block of code until the specified condition evaluates to false.
Common types of loops include for
, while
, and do-while
loops in many programming languages.
Loop statement works as follow diagram:
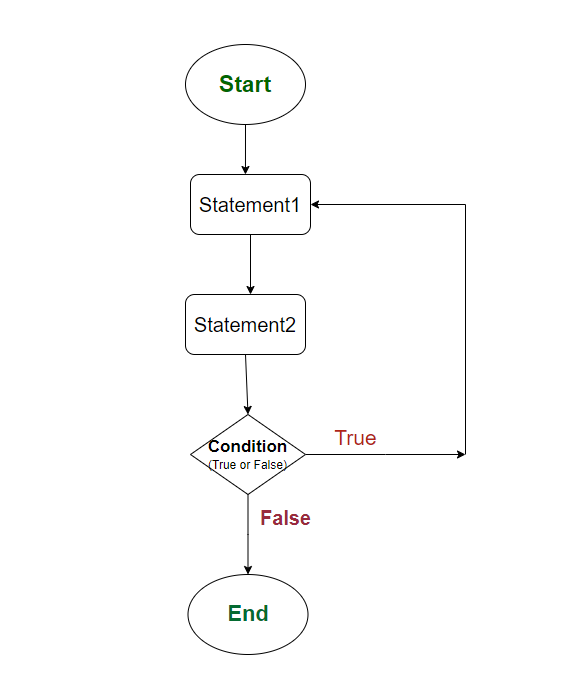
C language provides various loop statements that can form different forms of loop structures. The purpose of a loop is to repeat the same code multiple times.
1. Loop Types
According to the position of the control statement in the program, loops are classified into two types:
- Entry-controlled (Pre-check) loops
- Exit-controlled (Post-check) loops
Pre-check loops – Entry-controlled loops check the condition before executing the loop body, also known as pre-check loops.
Post-check loops – Exit-controlled loops check the condition after executing the loop body, also known as post-check loops.
Attention:
- It is essential to define and specify the control conditions well; otherwise, the loop will execute endlessly.
- A loop that continuously executes the statements is called an infinite loop, and an infinite loop is also referred to as a “never-ending loop.”
Characteristics of an infinite loop:
- No termination condition.
- The termination condition is never satisfied.
C programming has four types of loops:
- for loop (Pre-check)
- while loop (Pre-check)
- do…while loop (Post-check)
- goto (Post-check)
2. while Loop
The while
loop is a control flow statement that allows a block of code to be repeatedly executed as long as a given condition is true.
Syntex
while (condition) { statements; // Code to be executed repeatedly as long as the condition is true }
2.1. How while Loop Works?
Here’s how it works step by step:
- Condition Check: The loop begins with the evaluation of a specified condition. If the condition is true, the code inside the loop is executed. If the condition is false initially, the code inside the loop is skipped, and the program moves to the next statement after the
while
loop.while (condition) { // Code inside the loop }
- Execution of Code Block: If the condition is true, the code block inside the
while
loop is executed. This block can contain one or more statements. - Condition Re-evaluation: After executing the code block, the condition is re-evaluated. If the condition is still true, the loop repeats, and the code block is executed again. If the condition is false, the loop is terminated, and the program continues with the statement following the
while
loop. - Iteration: Steps 2 and 3 are repeated in each iteration of the loop until the condition becomes false.
while loop works as following diagram
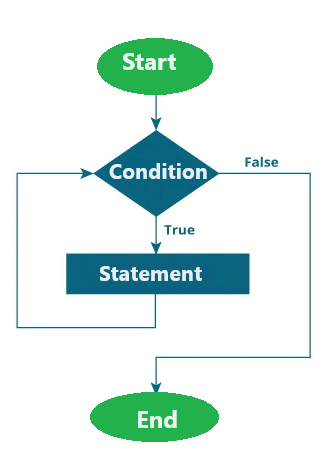
Example 2.1) a while
loop that prints numbers from 1 to 5
#include <stdio.h> int main() { int i = 1; while (i <= 5) { printf("%d ", i); i++; } return 0; }
Result:
1 2 3 4 5
Explanation:
- We initialize a variable
i
with the value 1. - The
while
loop continues to execute as long asi
is less than or equal to 5. - In each iteration, the value of
i
is printed, andi
is incremented by 1 (i++
). - The loop terminates when
i
becomes greater than 5.
Example 2.2 ) To calculate the sum from 1 to 100 using a while
loop
algorithm diagram for the sum from 1 to 100
code:
main() { int i=1,sum=0; while(i<=100) { sum=sum+i; i++; } printf("%d\n",sum); }
This program initializes a variable i to 1 and a variable sum
to 0. Then, it utilizes a while
loop to continually add the value of i to sum
while incrementing i until i exceeds 100.
The output should be the sum from 1 to 100.
Results:
asdgfdf