C identifiers are names used in a C program, such as variables, functions, arrays, structures, unions, labels, etc. Identifiers can be composed of letters, including uppercase and lowercase letters, underscores, and digits, but the first character must be a letter or an underscore. If an identifier is not used for external linkage, it is called an internal identifier. If an identifier is used for external linkage, it is called an external identifier.
We can say that an identifier is a collection of alphanumeric characters that starts with a letter character or an underscore and is used to represent various programming elements such as variables, functions, arrays, structures, unions, labels, etc. There are a total of 52 letter characters (uppercase and lowercase), the underscore character, and the ten digits (0-9) representing identifiers. There are a total of 63 alphanumeric characters (AZ, az), digits (0~9), and underscore (_) combined, and the first character must be a letter or an underscore, not a digit.
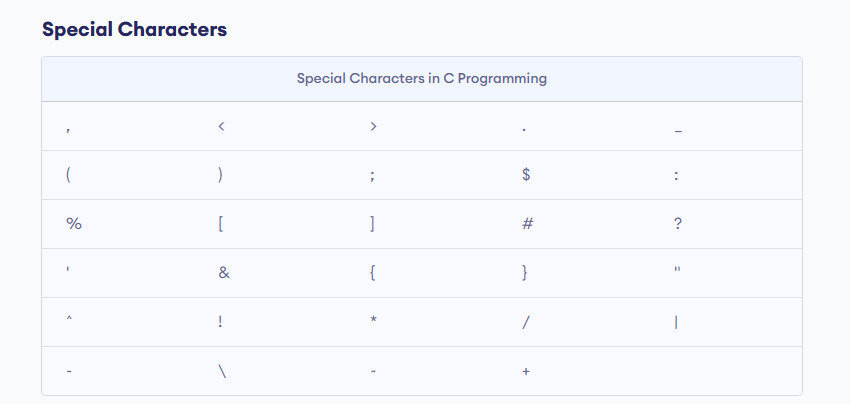
The Rules for Constructing C Identifiers are as Follows:
- The first character of an identifier should be a letter or underscore, followed by any character, digit, or underscore.
- After the first character, the identifier may contain any combination of letters, underscores, and digits (0 to 9).
- Reserved keywords such as “if”, “else”, “while”, and “for” cannot be used as identifiers.
- It cannot start with any digit.
- In identifiers, uppercase and lowercase letters are considered different. Therefore, identifiers are case-sensitive.
- Commas or whitespaces cannot be specified in identifiers. White space includes blank space, newline, horizontal tab, carriage return and form feed.
- Keywords cannot be used as identifiers.
- The length of an identifier cannot exceed 31 characters. The length of an identifier is not limited, but only the first 31 characters are significant. Identifiers that differ only in their 32nd character are considered identical.
- Identifiers cannot contain special characters such as punctuation marks or operators.
- It is considered good practice to choose descriptive and meaningful names for identifiers to make the code easier to read and understand.
Some Examples of Valid C Language Identifiers:
- count
- num_1
- total_sum
- is_valid
- studentName
- MAX_SIZE
- PI
- my_array
- struct_point
- function_name
Some Invalid C Language Identifiers:
Here are some examples of invalid C language identifiers:
- 1st_variable (Cannot start with a digit)
- hello world (Cannot contain spaces)
- sum, total (Cannot contain commas)
- #define (Is a keyword)
- my-variable (Cannot contain hyphens)
- long_identifier_name_exceeding_31_characters (Exceeds the length of 31 characters)
- 3s ( Can not start with a number )
- int (Reserved word)
- -3x ( Cannot start with” -” or “+”)
- bowy-wer ( Can not contain “-” inside the Identifier )
The Difference Between Identifiers and Keywords
an identifier is a user-defined name that represents a variable, function, or other programming element. On the other hand, a keyword is a predefined word with a specific meaning in the programming language.
Identifiers are created by programmers and must follow certain rules, such as starting with a letter or underscore, being unique within a scope, and not exceeding a certain length. Keywords, on the other hand, are part of the language’s syntax and have special meanings that cannot be altered by programmers.
For example, in C, “if”, “for”, “while”, and “return” are keywords that have predefined meanings in the language. On the other hand, “my_variable”, “sum”, “total”, and “function_name” are identifiers that are created by the programmer to represent variables or functions in the code.
Identifier | Keyword | |
---|---|---|
Definition | User-defined name that represents a variable, function, or other programming element | Predefined word with a specific meaning in the programming language |
Creation | Created by programmers | Part of the language’s syntax |
Rules | Must follow certain rules, such as starting with a letter or underscore, being unique within a scope, and not exceeding a certain length | Have predefined meanings that cannot be altered by programmers |
Examples | my_variable, sum, total, function_name | if, for, while, return |
An Example of Identifizer
<pre class="EnlighterJSRAW" data-enlighter-language="c">int main() { int a=10; int A=20; printf("Value of a is : %d", a); printf("\nValue of A is :%d", A); return 0; }</pre>
The output above shows that the values of the variables “a” and “A” are different. Therefore, we can conclude that C language identifiers are case-sensitive. In this example, even though the variables “a” and “A” have the same name, they are considered as two different identifiers because of their different cases.
This means that when writing C programs, it is important to pay attention to the case of identifiers to ensure correctness. If the same identifier with different cases is used in a program, it will be considered as two different identifiers.