The Basic Syntax of C we will study includes:
- Semicolon(;) and Whitespace
- Keywords
- Tokens
- Identifier
- Comments
- Header Files
1.Semicolon(;) and Whitespace
1.1 Statement
The code in the C language is composed of lines of statements.
A statement is a command that the program executes. A statement is a complete instruction that performs a specific action in a program. A statement can consist of one or more expressions, keywords, and other elements, and it usually ends with a semicolon (;).
Some examples of statements in C include:
- Assignment statements: These statements assign a value to a variable. For example,
x = 10;
assigns the value 10 to the variablex
. - Control statements: These statements control the flow of execution in a program. Examples include
if
statements,for
loops, andwhile
loops. - Function call statements: These statements call a function and pass arguments to it. For example,
printf("Hello, world!");
calls theprintf()
function to print the string “Hello, world!” to the console. - Declaration statements: These statements declare a variable and optionally initialize it with a value. For example,
int x = 10;
declares a variablex
of typeint
and initializes it with the value 10.
In C programming, a statement is generally executed in sequence, one after the other, unless a control statement changes the normal flow of execution. Understanding how to write and use statements effectively is essential for creating correct and efficient C programs.
1.2 Semicolon(;)
In C language, it is required that statements end with a semicolon(;) unless there are explicit rules that allow for omitting the semicolon (;).
int x = 1;
The above is a variable declaration statement, which declares an integer variable named x and initializes its value to 1.
Multiple statements can be written on a single line.
int x; x = 1;
The example above shows two statements written on a single line. Therefore, the newline character between the statements is not required; it is simply there for readability.
A single statement can also be written on multiple lines, in which case the semicolon (;) is used to indicate the end of the statement.
int x; x = 1 ;
In the example above, the second statement x = 1; was split into four lines, but the compiler will automatically ignore the newline characters in the code. In this case, the newline characters are only there to make the code more readable and do not affect the compiler’s ability to parse them as a single statement.
In fact, as long as the statement has a semicolon at the appropriate position within the same line, it can be split into multiple lines.
;
a single semicolon above can be a valid statement, which is called a “null statement” or “empty statement”.
Although it has no effect, it can be useful in certain situations, such as in a loop where only the condition needs to be checked and no actual statement is required in the loop body:
while(condition); // null statement used in the loop body
This will cause the loop to execute repeatedly while the condition is true, but since there is no actual statement inside the loop body, nothing will happen other than the condition being checked.
1.3 Expression
An expression is a combination of one or more constants, variables, operators, and function calls that are evaluated to produce a value. An expression can be as simple as a single variable or constant, or it can be a more complex combination of these elements.
1 + 2
The above code is an expression used to obtain the result of the arithmetic calculation “1 + 2”. In the C language, expressions and statements are different concepts. An expression is a calculation consisting of one or more operands and operators that produces a value. A statement is a complete unit of performing some kind of operation or semantic action, typically consisting of one or more expressions and terminated with a semicolon. Therefore, when an expression is followed by a semicolon, it becomes a statement, but it does not have any actual effect, because it does not perform any useful operation.
In actual program development, we typically use expressions to calculate certain values and assign them to variables or pass them to functions, etc. Statements are used to control the program’s execution flow, such as conditional judgments, loop control, etc. Understanding the concepts of expressions and statements and their applications in programs is essential for writing correct and efficient code.
8;
3 + 4;
The above example consists of two expressions that become statements after adding a semicolon at the end.
The main differences between expressions and statements are as follows:
- A statement can contain one or more expressions, but an expression itself does not constitute a statement.
- Expressions always have a return value, while statements do not necessarily have one. Statements are used to execute a command, and in many cases, they do not need to return a value. For example, a variable declaration statement such as
int x = 1
does not have a return value.
Understanding the difference between expressions and statements is important in programming, as it affects how we structure and organize our code. By using expressions and statements effectively, we can write clear and concise code that is easy to read and maintain.
Expressions in C can be categorized into several types, including:
- Arithmetic expressions: These expressions involve arithmetic operators such as addition (+), subtraction (-), multiplication (*), and division (/) to perform mathematical calculations.
- Relational expressions: These expressions involve relational operators such as less than (<), greater than (>), and equal to (==) to compare two values and produce a Boolean result (true or false).
- Logical expressions: These expressions involve logical operators such as logical AND (&&), logical OR (||), and logical NOT (!) to perform Boolean logic operations.
- Conditional expressions: These expressions use the ternary operator (?:) to select one of two values based on a condition.
- Bitwise expressions: These expressions involve bitwise operators such as bitwise AND (&), bitwise OR (|), and bitwise XOR (^) to perform bitwise operations on integers.
Expressions can appear in many places in a C program, including function calls, assignments, control structures, and more. They are a fundamental concept in programming and are used extensively in creating algorithms and data processing logic.
1.4 Whitespace
whitespace refers to any sequence of characters that represent horizontal or vertical space in the source code but do not affect the program’s functionality. Whitespace characters include spaces, tabs, and newline characters.
Whitespace is used in C to improve the code’s readability and structure. If syntax units can be distinguished without whitespace, whitespace is not necessary, but it is used to increase the readability of the code.
<pre class="EnlighterJSRAW" data-enlighter-language="c">int x = 1; // equals to int x=1;</pre>
In the example above, it is optional to have whitespace before or after the assignment operator (=) because the compiler can distinguish the syntax units without whitespace.
Multiple whitespace characters between syntax units are equivalent to a single whitespace character.
<pre class="EnlighterJSRAW" data-enlighter-language="c">int x = 1;
In the example above, multiple whitespace characters between syntax units have the same effect as a single whitespace character. Lines that contain only whitespace are called blank lines, and the compiler will completely ignore them. Whitespace is also used to indicate indentation. The compiler does not care whether the code is indented or not, as the code without indentation is still executable. Emphasizing code indentation is only to enhance the code readability and to distinguish between code blocks. 1.4.1 Indentation Most C language style guides require that the next level of code should be indented 4 spaces more than the previous level. It is common to use indentation to indicate the level of nesting in control statements like if
statements and loops. Here’s an example of code with and without indentation: Without indentation:
if (x > 0) {
printf("x is positive.");
}
With indentation:
if (x > 0) {
printf("x is positive.");
}
As you can see, the second example is much easier to read and understand because the indentation makes it clear which statements are nested inside the if block.
Whitespace is generally ignored by the compiler and does not affect the program’s behavior, except in some cases where whitespace is significant, such as in string literals and character constants.
It’s important to use whitespace effectively in your code to make it more readable and easier to understand for yourself and others who may need to read or modify it in the future.
1.5 A Statement Block
In C language, a statement block is a group of multiple statements enclosed in a pair of curly braces {}. It is treated as a single independent unit of statement. Statement blocks can appear wherever a statement is required, such as in if statements, while loops, for loops, etc., or can be independent. Statements within a block are executed in order and can contain other statement blocks.
The main purpose of statement blocks is to organize multiple statements into a single logical unit, making it easier to control program flow, improve code readability, and maintainability. Additionally, statement blocks are often used to define local variables, which are only visible within the block and are destroyed when the block is left.
Here is an example program that demonstrates how to use statement blocks:
#include <stdio.h> int main() { int x = 1; { int y = 2; printf("x + y = %d\n", x + y); } // y is not visible here, it's out of scope printf("x = %d\n", x); return 0; }
In the above program, we define a statement block that includes a local variable y and an output statement. Inside the statement block, we can access both x and y variables, but outside the block, the y variable is no longer visible. This effectively isolates the scope of variables, avoids unnecessary naming conflicts, and improves program reliability.
2. Tokens
A C program consists of various tokens and a token is either a keyword, an identifier, a constant, a string literal, or a symbol. A token is a basic unit of syntax that the compiler or interpreter uses to understand the structure and meaning of a program.
A token is a sequence of characters that represents a single element of the program, such as a keyword, identifier, operator, a constant, a string literal, a symbol, or punctuation symbol. .
For example, in the C programming language, the statement
int x = 10;
contains four tokens:
- “int” is a keyword that indicates the variable’s data type.
- “x” is an identifier that names the variable.
- “=” is an operator that assigns the value 10 to the variable.
- “10” is a constant that represents the value assigned to the variable.
The compiler uses these tokens to analyze the program’s syntax and semantics and generate the corresponding executable code.
In general, tokens are the building blocks of a program’s syntax, and understanding how they work is crucial to writing correct and efficient code.
Here is a table of the different types of tokens in the C programming language:
Token Type | Description |
---|---|
Identifiers | Names given to variables, functions, and other program elements. |
Keywords | Reserved words that have a specific meaning in the C language. |
Constants | Fixed values that do not change during program execution. |
Operators | Symbols that perform specific mathematical or logical operations. |
Punctuators | Symbols that are used for grouping and separating program elements. |
String literals | A sequence of characters enclosed in double quotes. |
Character literals | A single character enclosed in single quotes. |
Comments | Text in a program that is ignored by the compiler, used for documentation. |
Directives | Special instructions to the compiler that are preceded by a pound (#) sign. |
Examples of each token type in C:
Token Type | Example |
---|---|
Identifiers | count, x, sum |
Keywords | if, while, for, int, void |
Constants | 42, 3.14, ‘a’, “Hello, world!” |
Operators | +, -, *, /, %, =, ==, >, <, &&, |
Punctuators | ;, ,, ., :, (, ), {, }, [, ] |
String literals | “Hello, world!”, “The quick brown fox jumped over…” |
Character literals | ‘a’, ‘7’, ‘\n’, ‘\t’ |
Comments | /* This is a comment */ |
Directives | #include |
Note that some tokens, such as constants and operators, can have subcategories depending on the type of constant (integer, floating-point, etc.) or the specific operator (addition, subtraction, etc.).
3. Keywords
Here is a table of commonly used keywords in the C programming language:
Keyword | Description |
---|---|
auto | Declares a local variable with a limited scope. |
break | Terminates a loop or a switch statement. |
case | Used in a switch statement to define a specific condition. |
char | Defines a character data type. |
const | Defines a read-only variable. |
continue | Used in a loop to skip to the next iteration. |
default | Specifies a default case in a switch statement. |
do | Starts a do-while loop. |
double | Defines a floating-point data type. |
else | Defines an alternative case in an if-else statement. |
enum | Defines a custom data type with named values. |
extern | Declares a variable that is defined in another file. |
float | Defines a floating-point data type. |
for | Starts a loop with a specified condition. |
goto | Transfers program control to a labeled statement. |
if | Starts a conditional statement. |
int | Defines an integer data type. |
long | Defines a long integer data type. |
register | Suggests to the compiler to store a variable in a register. |
return | Returns a value from a function. |
short | Defines a short integer data type. |
signed | Defines a signed integer data type. |
sizeof | Returns the size of a data type or variable. |
static | Declares a variable with a fixed size and persistent scope. |
struct | Defines a custom data type with multiple elements. |
switch | Starts a switch statement. |
typedef | Defines a custom data type. |
union | Defines a custom data type with overlapping elements. |
unsigned | Defines an unsigned integer data type. |
void | Specifies that a function does not return a value. |
volatile | Specifies that a variable may be modified outside the program. |
while | Starts a while loop. |
Note that some of these keywords, such as auto and register, are rarely used in modern C programming and have been deprecated in newer versions of the language.
4. Identifier
an identifier is a name given to a variable, function, or any other user-defined item. An identifier is a sequence of letters (both uppercase and lowercase), digits, and underscore characters (_). It must begin with a letter or an underscore.
Here is a table of C identifiers and their allowable characters:
Identifier Type | Allowable Characters |
---|---|
Variables | letters, digits, and underscore character; must begin with a letter or underscore |
Functions | letters, digits, and underscore character; must begin with a letter or underscore |
Constants | letters, digits, and underscore character; typically written in all uppercase letters |
Labels | letters, digits, and underscore character; must begin with a letter or underscore |
Note that C is a case-sensitive language, which means that uppercase and lowercase letters are considered distinct. Therefore, the identifier “foo” is different from “FOO” or “Foo”.
Also, it is important to choose meaningful and descriptive names for identifiers to make the code more readable and understandable. Avoid using reserved keywords as identifiers, as they have special meanings in the language and cannot be used as variable names.
5. Comments
Comments are explanations for the code, and the compiler will ignore comments, which means that comments have no effect on the actual code. There are two ways to represent comments in the C language. The first method is to enclose the comment in /*…*/, and it can be spread across multiple lines.
<pre class="EnlighterJSRAW" data-enlighter-language="c">/* Comments */ /* Comments */</pre>
This type of comment can also be inserted inline.
int open(char* s /* file name */, int mode);
In the example above, /* file name */ is used to explain the function parameter, and the code that follows it will still be executed. It is important to remember to include the ending symbol */ in this type of comment, otherwise it can easily lead to errors.
<pre>rintf("a "); /* comment1 printf("b "); printf("c "); /* comment2 */ printf("d ");
The meaning of the example above is that there are two comments at the end of the first and third lines of code. However, the comment in the first line forgot to write the closing symbol, which caused the comment to extend to the end of the third line.
//comments
The second way to write comments in C is to use double slashes // followed by the comment text. The text following the double slashes until the end of the line is considered as a comment. This type of comment can only be a single line and can be placed at the beginning of a line or at the end of a statement. This syntax was introduced in the C99 standard.
printf("// hello /* world */ ");
Regardless of the type of comment, it should not be placed within double quotes. Comment symbols inside double quotes are treated as regular characters and lose their commenting effect. In the example given, the comment symbols inside the double quotes will be treated as ordinary characters and will not be treated as comments by the compiler.
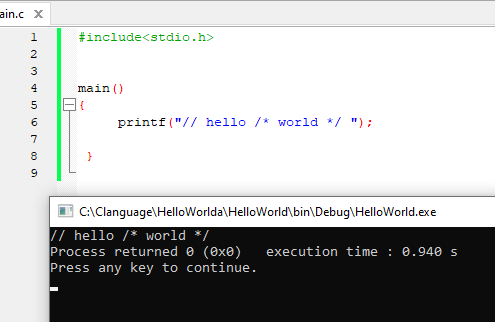
6. Header Files
The standard library in C language provides a collection of functions, macros, and constants that are widely used in programming. These functions are included in the header files of the standard library, which can be included in a C program using the #include directive.
Standard header files are named with a “.h” extension, and they typically contain declarations for functions, macros, and constants related to a specific area of functionality. For example, the <stdio.h> header file contains functions for input and output operations, while the <stdlib.h> header file contains functions for memory allocation and other general-purpose functions.
To use a function from the standard library, you need to include the appropriate header file in your program and then call the function using its name. The standard library functions are well-documented and widely used, making them a valuable resource for C programmers.
Programmers can simply call these built-in functions to avoid writing their own code. For example, the printf() function is built into the C language, and by calling it, you can output content on the screen.
Different functions are defined in different files, and these files are collectively referred to as “header files.” If a system includes a certain function, it will also include the header file that describes that function. For example, the header file for printf() is stdio.h, which is included in the system. The suffix for header files is usually .h.
To use a function, you must first load the corresponding header file using the #include command. This is why you must load stdio.h before using printf().
6.What is the Difference Between Expression and Statement ?
In C, an expression is a combination of operators and operands that evaluate to a single value, while a statement is a complete line of code that performs some specific action, such as assigning a value to a variable or calling a function.
Here are some key differences between expressions and statements in C:
- An expression always produces a value, while a statement may or may not produce a value.
- An expression can be used as part of a statement, but a statement cannot be used as part of an expression.
- An expression can be composed of one or more operands and operators, while a statement is usually composed of one or more expressions and a semicolon (;) to indicate the end of the statement.
- Expressions can be nested within other expressions, while statements can be nested within other statements.
- An expression can be assigned to a variable, while a statement cannot be assigned to anything.
Here’s an example to illustrate the difference between expressions and statements:
int x = 2 + 3; // This is a statement that assigns the value of 5 to the variable x int y = (x > 3); // This is an expression that evaluates whether x is greater than 3 and returns a value of 1 (true) or 0 (false) if (y == 1) { // This is a statement that executes the code within the if block if the condition (the expression y == 1) is true printf("x is greater than 3"); }
In the example above, we can see that expressions and statements are both important building blocks of C programming, but they serve different purposes.
Expressions are used to evaluate values, while statements are used to perform actions and control program flow.