Before learning about the program structure of C language, let’s first take a look at a simple program.
Input the three sides of a triangle and calculate its area.
Given the three sides of a triangle a, b, and c, the formula for calculating the area of the triangle is:
1. The Simple Program Of C Programming Language
Source code of above mathematical formula:
#include<math.h> #include<stdio.h> main() //main function { float a,b,c,s,area; //Declare four floating-point numbers scanf("%f %f %f",&a,&b,&c); //Enter the three side lengths of the triangle s=1.0/2*(a+b+c); /* The formula for calculating the area of a triangle. The calculation process follows a mathematical formula. */ area=sqrt(s*(s-a)*(s-b)*(s-c)); printf("a=%f,b=%f,c=%f,s=%f\n",a,b,c,s); //Print the three side lengths and s formatted printf("area=%7.2f\n",area); // Print the area of the triangle formatted }
Let’s explain above source code :
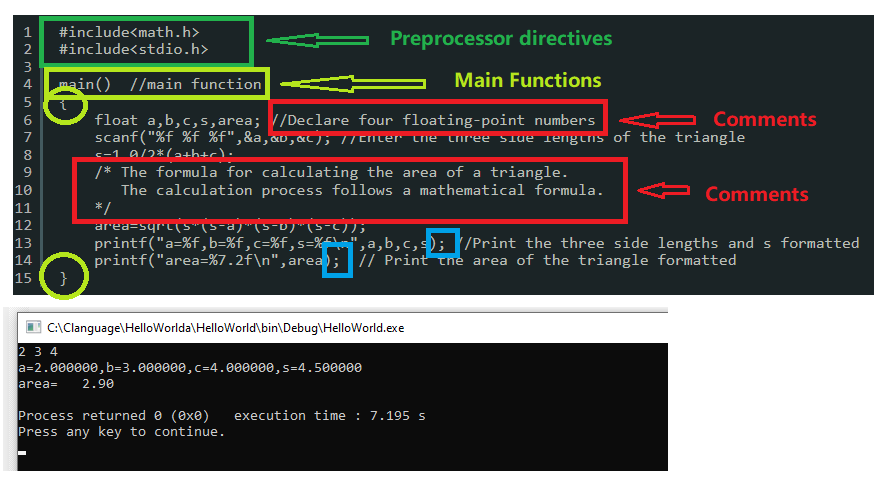
2.The Program Structure of C Program language
We can see the program structure of C language through the above program. Here we focus more on the program structure rather than the program itself.
- Preprocessor directives
- Functions
- Variables
- Statements & Expressions
- Comments
2.1 Preprocessor Directives (Header and Macro)
In order to use the printf() function provided by the standard IO library, we used the statement “# include <stdio.h>”, which represents that we want to use the functions provided by the STDIO library in the program. We also call files such as stdio.h header files. If we use mathematical functions, we need the math.h header file.
The header file mainly contains function declarations. This makes it convenient for us to reuse programs that others have already implemented. If we can use others’, we generally don’t need to implement our own. These are actually called C library functions. Library functions are built-in functions that are grouped together for C program preprocessor to call when the C programmer uses them.
All C standard library functions are declared by using many header files. These library functions are created at the time of designing the compilers. The preprocessor directive will guide the compiler to find the content we need to reference during program compilation.
2.2 Main Function (main body of the program)
Every C program needs to include a main function. This is the main body of the program, and every executable C program must have a main function as the entry point of the program.
The pair of parentheses ( ) after the main function, the pair of curly braces { }after the parentheses, and the set of program code contained within the braces { } make up the main body of the program.
The parentheses ( ) can contain parameters that need to be passed to the main function when the program starts. It can be one or more parameters. The part within the curly braces { } is the main body of the program. It contains executable program statements or related content such as comments.
2.3 Variable Declaration
float a, b, c, s, area
The above program is variable declaration. Variables a, b, c, s, and area are declared as float variables. In C language, variables need to be declared with their types before they can be used. This is a bit different from some other programming languages. Different variable types in C language correspond to different memory allocation sizes.
2.4 Statements and Expressions
In addition to the parts discussed above, the remaining parts are program statements. The printf() function outputs formatted text to the standard terminal, while the scanf() function inputs formatted text from the standard terminal.
Statements are generally a combination of functional functions and conditional structures. The program will perform corresponding operations based on the change of conditions, and eventually calculate the results we need.
2.5 Comments in C language
In C language, comments are used to add notes or explanations to the code that are not executed by the computer. They are used to help developers understand the purpose and functionality of a particular section of code.
There are two types of comments in C:
- Single-line comments: These comments begin with two forward slashes (//) and extend to the end of the line. They are used to comment on a single line of code.
- Multi-line comments: These comments begin with a forward slash and an asterisk (/*) and end with an asterisk and a forward slash (*/). They are used to comment on multiple lines of code.
Here’s an example of how comments can be used in C code:
#include <stdio.h> int main() { // This is a single-line comment /* This is a multi-line comment */ printf("Hello, World!"); // This is another single-line comment return 0; }
3. Summary
The program structure of C language typically includes the following parts:
- Header section: Use the
#include
directive to import the required header files, such asstdio.h
,stdlib.h
, etc. - Macro definition section: Use the
#define
directive to define constants and macros, such as#define PI 3.14159
, etc. - Global variable section: Define global variables that can be accessed throughout the program.
- Function declaration section: Use function prototypes to declare the functions that need to be used so that they can be called in the main function.
- Main function section: The entry function of the program, which contains the main body of the program.
- Custom function section: Contains custom functions that are used to perform specific functions.
The basic structure of a C language program is shown as follows:
#include <stdio.h> /* Header section */ #define PI 3.14159 /* Macro definition section */ int global_var; /* Global variable section */ void function1(int arg1, float arg2); void function2(); /* Function declaration section */ int main() { /* Main function section */ // ... return 0; } void function1(int arg1, float arg2) { // ... } void function2() { // ... } /* Custom function section */
Note that the program structure of C language is not fixed, and different programming styles and project requirements may vary.