Integers are typically stored in memory using a fixed number of bits, depending on the size of the integer. For example, a 32-bit integer can store values from -231 to 231-1.
The binary representation of the integer is stored in memory using these bits, with the most significant bit representing the sign of the integer (0 for positive, 1 for negative) and the remaining bits representing the magnitude of the integer.
The process of storing integers in memory is handled by the computer’s central processing unit (CPU), which can perform arithmetic and logical operations on the bits representing the integers. The CPU also includes registers, which are small, fast storage locations used to hold frequently accessed data, including integer values.
When a program accesses an integer value, the CPU retrieves the bits representing the integer from memory and stores them in one of its registers, where it can perform calculations on the integer. Once the calculation is complete, the CPU stores the result back in memory as a series of bits, which can be read and interpreted by the program.
In the C language, the value of an integer variable can be expressed in decimal, octal, or hexadecimal format, but it is stored in memory as a binary number.
1.The Storage Method of Positive Integers in Computers
For example, to convert decimal 65 to binary, first convert it into binary number as 1000001. For specific methods of converting decimal to binary, please refer to this link.
When we declare a integer variable
int i; i=65;
The memory will arrange a 32 bit memory to store the positive integer in following method:
The sign bit is also called the MSB (most significant bit). If the sign bit is 0, it is a positive integer. If a sign bit is 1, it is a negative integer.
2.The Storage Method of Negative Integers in Computers
Computer uses special mechanism to store negative numbers which is 2’s complement format.
Before going to that, let’s have a look on 1’s complement of a number.
1’s complement of a number
1’s compliment of number is just inverting binary bits of an actual number.
2’s complement of a number
To get 2’s complement of a number, just add 1 to 1’s complement of an actual number.
This 2’s complement form will be stored in computer.
Both one’s complement and two’s complement can not change the sign bit (MSB)
When we declare a negative integer variable,
int i; i=-10;
The memory will assign above memory for -10 ( 16 bit system )
3. The Character Data Type and Character Variable
3.1 What is Character Data Type ?
In C programming, the character data type is a primitive data type used to store a single character. The character data type is represented using the keyword ‘char’ and is typically one byte in size. It can store characters, such as letters, digits, punctuation marks, and other special characters.
A character variable is a variable used to store a value of the character data type. It is declared using the ‘char’ keyword, followed by the variable name. For example, to declare a character variable named ‘myChar’ and assign it the value ‘A’, you can use the following code:
3.1.1 Syntax:
char myChar = 'A';
In the above code, ‘myChar’ is the variable name, ‘char’ is the data type, and ‘A’ is the value assigned to the variable.
Character variables are commonly used in C programming for various purposes, such as input/output operations, string handling, and other applications that require character manipulation.
3.1.2 The Memory of char Data Type
Characters are stored in 8 bits or 1 byte of memory and can be either signed or unsigned. There is no separate “character type” in the C language. The ‘char’ type is essentially the smallest integer type, and as such, it can be signed or unsigned, just like any other integer type.
How many memory storage for a char Data Type.
- char 1 byte −128 to 127
- signed char 1 byte −128 to 127
- unsigned char 1 byte 0 to 255
Both signed and unsigned characters are stored as a single character based on their ASCII value. For example, the character ‘A’ will be stored as 65. We do not need to specify the keyword ‘signed’ to use a signed char in C programming language.
//Both are same signed char name = ‘a’; char name = ‘a’;
n C programming language, we need to use the ‘unsigned’ keyword to specify an unsigned character. If the ‘unsigned’ keyword is not used, the character is assumed to be signed by default.
For example, to declare an unsigned character variable named ‘myUnsignedChar’ and assign it the value ‘B’, you can use the following code:
unsigned char myUnsignedChar = 'B';
In the above code, ‘myUnsignedChar’ is the variable name, ‘unsigned char’ is the data type, and ‘B’ is the value assigned to the variable.
3.1.3 Character Input and Output
the character data type is stored using one byte (8 bits) in computers. In C programming language, characters are treated as integers, so the character data type is essentially an integer with a width of one byte. Each character corresponds to an integer value (determined by its ASCII code), such as ‘B’ corresponds to the integer 66.
The default range for character data type varies across different computer systems. Some systems use a range of -128 to 127, while others use a range of 0 to 255. Both ranges can cover the ASCII character range from 0 to 127.
The ASCII table contains 128 character values from 0 to 127, while the remaining 127 characters are referred to as the extended ASCII character set.
Example 3.1 Characters are Input and Output as Integers
char c = 66; // equals to
char c = 'B';
As long as the integer value is within the range of the character data type, integers and characters can be interchanged and assigned to variables of the character data type. In the example above, the variable ‘c’ is of the character data type and assigned an integer value of 66. This is equivalent to assigning the character ‘B’ to the variable ‘c’.
#include <stdio.h> #include <stdlib.h> int main() { char a = 'B'; char b = 66; printf("a=%d|b=%d\n", a,b); return 0; }
Example 3.2. Characters Input as Integers and Output as Characters
#include <stdio.h> #include <stdlib.h> int main() { char a = 'B'; char b = 66; printf("a=%c|b=%c\n", a,b); return 0; }
3.2 Mathematical Operations Between two Variables of Character Data Type
char a = 'B'; // equals to : char a = 66; char b = 'C'; // equals to : char b = 67; printf("%d\n", a + b); // Output: 133
In the above example, adding two character variables a and b is treated as adding two integers. The placeholder %d is used to print the decimal integer value of the result, which is 133.
In the signed character extended set, the values range from -128 to -1, while in the unsigned character it ranges from 128 to 255. When you try to assign a value of 128 to a signed character, it will wrap around like a clock and take the value of -128.
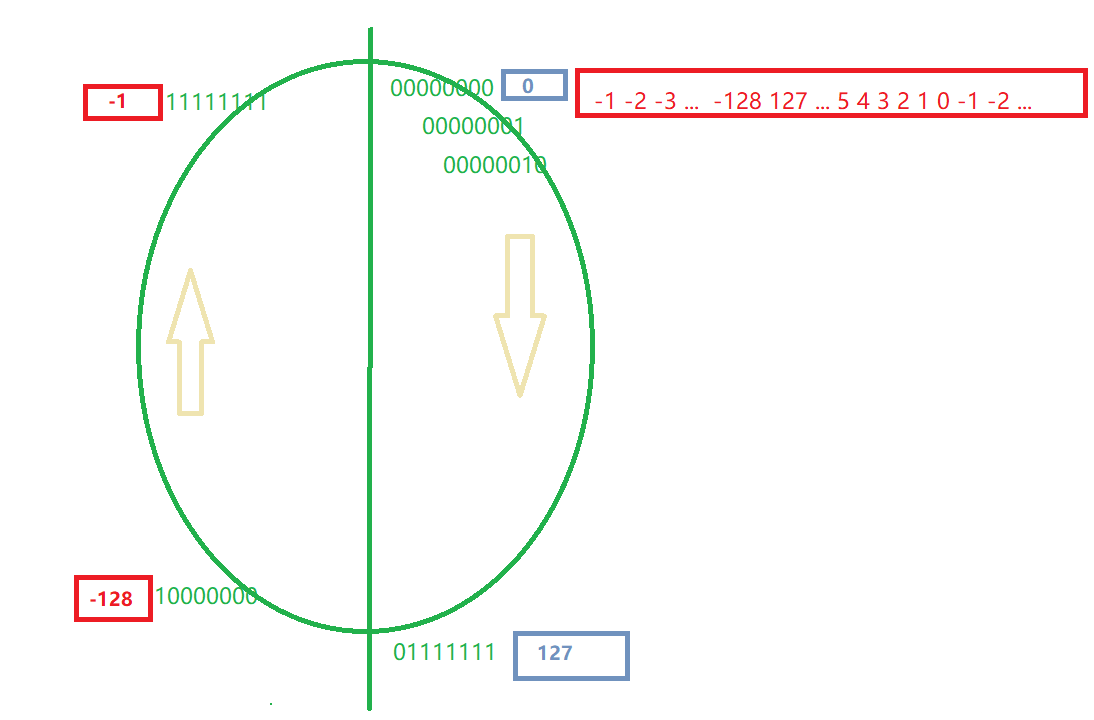
Similarly, if you assign a value of 256 to an unsigned char, it will wrap around and take the value of 0.
Example 3.3 Overflow of unsigned char.
#include <stdio.h> #include <stdlib.h> int main() { unsigned char a = 256; printf("chartype a=%c\n", a); printf("integertype a=%d\n", a); return 0; }