1. Character Literal
A character literal is a single character enclosed in English single quotes (”). When using character literals, the following should be noted:
- Uppercase and lowercase characters within the single quotes represent different character literals. For example, ‘Y’ and ‘y’ are two different character literals.
- Character literals must be enclosed in single quotes(”), not double quotes(“”). For example, “Y” is not a character constant, it is a string.
- If a space character is within the single quotes, it is also a character literal.
- Only one character can be included within the single quotes. The notation ‘xyz’ is incorrect. However, if more than one character is included, all characters except the last one will be automatically invalidated. This should be avoided when programming.
- The value of a character literal is its corresponding integer value in the ASCII encoding table. It is an integer between 0 and 127.
Therefore, a character literal can be treated as an integer data type and used in mathematical operations.
For example:
- The value of the expression ‘Y’ + 32 is 121, which is the value of ‘y’.
- The value of the expression ‘7’ + ‘6’ is 109, which corresponds to the character ‘m’ in the ASCII table.
- It should be noted that ‘7’ and 7 are different. ‘7’ as a character literal represents an integer constant value of 55, while 7 as an integer constant represents the integer constant value of 7.
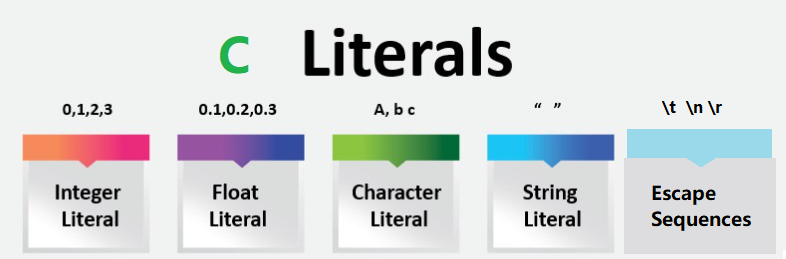
2. Escape Sequences
An escape sequence is a combination of characters that represents a special character, which cannot be represented directly in a string or character literal.
In C programming language, there are 256 numbers of characters in character set. The entire character set is divided into 2 parts i.e. the ASCII characters set and the extended ASCII characters set.
But apart from that, some other characters are also there which are not the part of any characters set, known as ESCAPE characters.
Escape sequences are used to represent special characters in strings or characters, such as the newline character (\n), the tab character (\t), the backslash character (\), and the null character (\0), among others. These characters are considered special because they cannot be typed directly on the keyboard or represented in a character or string literal.
Escape sequences are represented by a backslash () followed by a character or a combination of characters. For example, the escape sequence for the newline character is \n, and the escape sequence for the tab character is \t.
Escape sequences are widely used in C programming language to format output, print special characters, and manipulate strings and characters in various ways.
here’s a table with some of the commonly used escape sequences in C:
Escape Sequence | Description |
---|---|
\n | newline character |
\t | tab character |
\r | carriage return character |
\b | backspace character |
\f | form feed character |
\v | vertical tab character |
‘ | single quote character |
“ | double quote character |
\ | backslash character |
\0 | null character |
Note that the escape sequence for each special character is represented by a backslash (\) followed by a specific character or combination of characters.
escape sequences we frequently use:
\a
: Alert, this will cause the terminal to emit a warning sound or flash, or both.
\b
: Backspace key, the cursor moves back one character, but the character is not deleted.
\f
: Form feed, the cursor moves to the next page. On modern systems, this behavior is similar to \v.
\n
: Newline character.
\r
: Carriage return, the cursor moves to the beginning of the same line.
\t
: Tab character, the cursor moves to the next horizontal tab position, usually the next multiple of 8.
\v:
Vertical tab character, the cursor moves to the next vertical tab position, usually the same column on the next line.
\0:
Null character, represents no content. Note that this value is not equal to the number 0.
Escape notation can also use octal and hexadecimal to represent a character.
\nn:
The octal notation for a character, where nn is an octal value.
\xnn:
The hexadecimal notation for a character, where nn is a hexadecimal value.
char x = 'B'; char x = 66; char x = 0102; // octal value char x = '\x42'; // hexadecimal value
The four forms of notation shown above are equivalent.
Example 2.1 Application of Escape Characters
#include <stdio.h> #include <stdlib.h> int main() { char a = 'B'; char b = 66; char c = 0102; char d = '\x42'; printf("chartype a=%d\n", a); printf("dectype b=%d\n", b); printf("octtype c=%d\n", c); printf("hextype d=%d\n", d); return 0; }
results: