1. Integers
In computer programming, an integer is a data type that represents a whole number. For example, 0, -5, 10. This means that an integer can only represent numbers without fractional or decimal parts. Integers can be positive, negative, or zero, and they can be represented using a finite number of bits in binary form.
In most programming languages, integers are represented using a fixed number of bits, such as 8, 16, 32, or 64 bits. The maximum and minimum values that can be represented by an integer depend on the number of bits used, with larger numbers of bits allowing for a greater range of values.
The size of an int is typically 4 bytes (32 bits). Integers are stored in a computer using 4 bytes (32 bits) of memory.
The value range of a 4-byte integer is -2147483648 to 2147483647.
For example:
int a = 456;
Now we convert 456 into binary digits:
1 0 1 1 0 0 1 0 0
Since int is allocated 32 bits, the remaining 23 bits are filled with 0s.
The 456 in memory :
00000000 00000000 00000001 01100100
2. How is the Integer Data Type classified ?
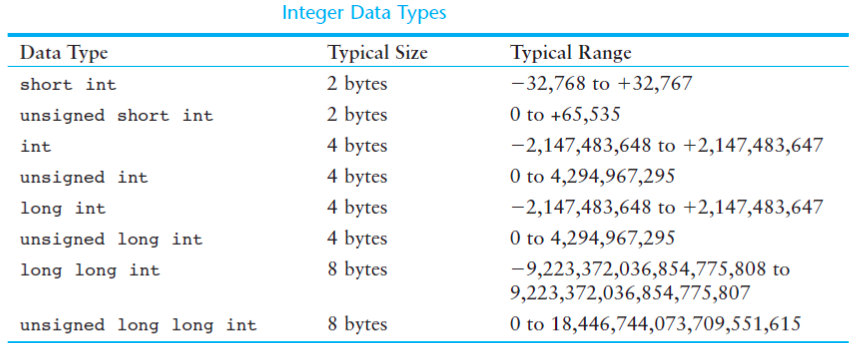
In computer programming, the integer data type can be classified into several types, typically including:
Short integer (short): Typically, a short integer uses 2 bytes (16 bits) of memory to store an integer value. The range of values that can be represented by a short integer varies depending on the programming language, but it is typically in the range of -32,768 to 32,767.
Integer (int): An integer typically uses 4 bytes (32 bits) of memory to store an integer value. The range of values that can be represented by an integer is much larger than that of a short integer, typically in the range of -2,147,483,648 to 2,147,483,647.
Long integer (long): A long integer typically uses 8 bytes (64 bits) of memory to store an integer value. The range of values that can be represented by a long integer is even larger than that of an integer, typically in the range of -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
Unsigned integer: An unsigned integer is a non-negative integer that does not have a sign bit, which means it cannot represent negative values. By not using a sign bit, the range of positive values that can be represented by an unsigned integer is doubled, but the range of values is shifted towards positive values.
Byte: A byte is the smallest unit of memory used to store data, typically consisting of 8 bits. In some programming languages, a byte can be used to represent small integer values in the range of 0 to 255.
The specific types of integer data types available in a programming language may vary, and the size and range of values that can be represented by each type may depend on the hardware architecture and programming language used.
3.Signed and Unsigned Integers
In C language, signed and unsigned are data type modifiers used to specify the range of integer types. Integer types that are modified with signed are considered signed integer types, and their value can be positive, negative, or zero. On the other hand, integer types modified with unsigned are considered unsigned integer types, and their value can only be non-negative (including zero), and therefore cannot represent negative numbers.
Signed and unsigned modifiers can be used for integer types such as char, short, int, long, long long, etc. to change the range of values of the integer type by changing their storage method. Specifically, integer types modified with signed use two’s complement representation to store data, which can represent positive numbers, negative numbers, and zero. On the other hand, integer types modified with unsigned use unsigned binary representation to store data, and can only represent non-negative numbers.
It should be noted that using the unsigned modifier can expand the range of integer types, but it can also bring some potential problems. For example, when performing arithmetic operations, if an unsigned integer type is used and the result of the operation is negative, it may cause overflow errors. Therefore, extra caution should be taken when using the unsigned modifier to avoid these types of errors.
For Example 3.1:
signed int a=2357;
its Binary format:
1 0 0 1 0 0 1 1 0 1 0 1
Because the computer assign 32 bit to an integer, 2357 is stored in memory in following format.
00000000 00000000 00001001 00110101
For example 3.2:
unsigned int x; int y;
Here, the variable x can only hold zero or positive values because we used the unsigned modifier. Considering that the size of an int is 4 bytes, the variable y can hold values from -2147483648 to 2147483647, while the variable x can hold values from 0 to 4294967295.