A JavaScript operator is a symbol or a keyword that is used to perform operations on one or more operands, such as variables, values, or expressions. Operators enable you to manipulate and work with data in your JavaScript code. They are classified into various categories based on the type of operation they perform.
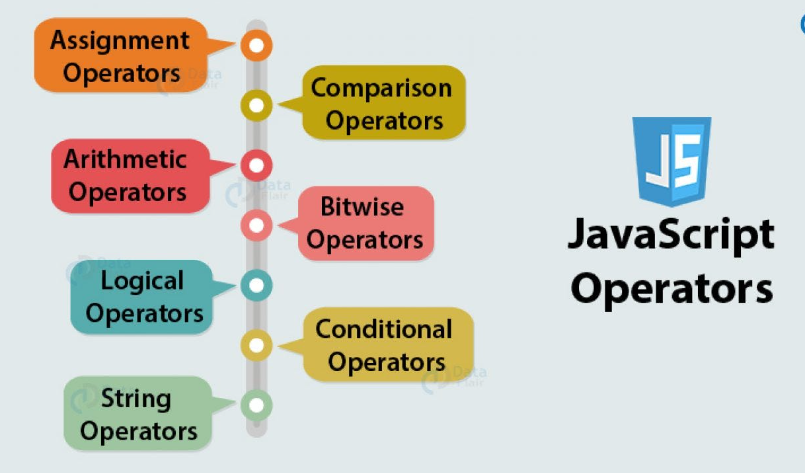
JavaScript operators closely resemble operators found in other programming languages. Operators, by definition, are symbols utilized to carry out operations. While these operations frequently involve arithmetic calculations, such as addition or subtraction, they can encompass a wide array of functions.
Here are some common categories of JavaScript operators:
- Arithmetic Operators: These operators are used for mathematical calculations.
- Addition
+
- Subtraction
-
- Multiplication
*
- Division
/
- Modulus (Remainder)
%
- Exponentiation
**
(in some versions of JavaScript)
- Addition
- Comparison Operators: These operators are used to compare values and determine the relationship between them.
- Equal to
==
or===
(strict equality) - Not equal to
!=
or!==
(strict inequality) - Greater than
>
- Less than
<
- Greater than or equal to
>=
- Less than or equal to
<=
- Equal to
- Logical Operators: Logical operators are used to perform logical operations on Boolean values.
- Logical AND
&&
- Logical OR
||
- Logical NOT
!
- Logical AND
- Assignment Operators: Assignment operators are used to assign values to variables.
- Assignment
=
- Add and assign
+=
- Subtract and assign
-=
- Multiply and assign
*=
- Divide and assign
/=
- Assignment
- Increment/Decrement Operators: These operators are used to increase or decrease the value of a variable.
- Increment
++
- Decrement
--
- Increment
- Conditional (Ternary) Operator: A shorthand way to write simple conditional statements.
condition ? expression1 : expression2
- Bitwise Operators: Bitwise operators perform operations at the binary (bit) level.
- Bitwise AND
&
- Bitwise OR
|
- Bitwise XOR
^
- Bitwise NOT
~
- Left shift
<<
- Right shift
>>
- Zero-fill right shift
>>>
- Bitwise AND
- String Operators: String operators are used to concatenate or manipulate strings.
- Concatenation
+
(also used for addition)
- Concatenation
- Type Operators: These operators check the data type or instance of an object.
typeof
(returns the type of a value)instanceof
(checks if an object is an instance of a specific class or constructor)
JavaScript operators are essential for performing a wide range of operations in your code, from simple arithmetic calculations to complex logical evaluations. Understanding how to use these operators effectively is fundamental to writing functional and expressive JavaScript programs.
Operator Precedence in JavaScript
Operator precedence in JavaScript determines the order in which operators are evaluated when an expression contains multiple operators. This is crucial because it ensures that expressions are evaluated correctly and consistently. JavaScript follows a set of rules to determine the precedence of operators, similar to how mathematics defines operator precedence. Operators with higher precedence are evaluated before operators with lower precedence.
Here is a summary of operator precedence in JavaScript, from highest to lowest:
- Grouping: Parentheses
()
. Expressions enclosed in parentheses are evaluated first. - Member Access: Dot notation (
.
) and bracket notation ([]
). Used to access object properties or array elements. - Function Call: Invoking a function or method using parentheses
()
. - New: Creating instances of objects using the
new
keyword. - Increment/Decrement:
++
(postfix) and--
(postfix). Increment or decrement operators used after a variable. - Unary Operators:
+
(positive),-
(negation),!
(logical NOT),~
(bitwise NOT),typeof
,void
, anddelete
. These operators have higher precedence than most binary operators. - Exponentiation:
**
. Raised to the power of. - Multiplication/Division/Modulus:
*
(multiplication),/
(division), and%
(modulus). These are binary operators for basic arithmetic. - Addition/Subtraction:
+
(addition) and-
(subtraction). Binary operators for addition and subtraction. - Bitwise Shift:
<<
(left shift),>>
(signed right shift), and>>>
(unsigned right shift). Used for bit manipulation. - Relational Operators:
<
,>
,<=
,>=
,instanceof
, andin
. Used for comparisons. - Equality Operators:
==
,!=
,===
, and!==
. Used for equality comparisons. - Bitwise AND:
&
. Bitwise AND operation. - Bitwise XOR:
^
. Bitwise XOR operation. - Bitwise OR:
|
. Bitwise OR operation. - Logical AND:
&&
. Logical AND operation. - Logical OR:
||
. Logical OR operation. - Conditional (Ternary) Operator:
? :
. Used for conditional expressions. - Assignment Operators:
=
,+=
,-=
,*=
,/=
,%=
,<<=
,>>=
,>>>=
,&=
,^=
, and|=
. Used for variable assignment.
Operators with higher precedence are evaluated first, and when operators have the same precedence, their evaluation follows a specific associativity (left-to-right or right-to-left) based on the operator.
In this table, 1 is the highest precedence and 19 is the lowest precedence.
Precedence | Operator | Description | Associativity | Example |
---|---|---|---|---|
1 | () | Grouping | – | (1+2) |
2 | . | Member | left to right | obj.function |
[] | Member | left to right | obj[“func”] | |
new | Create | – | new Date() | |
() | Function call | left to right | func() | |
3 | ++ | Postfix increment | – | i++ |
— | Postfix decrement | – | i– | |
4 | ++ | Prefix increment | right to left | ++i |
— | Prefix decrement | –i | ||
! | Logical NOT | !TRUE | ||
typeof | Type | typeof a | ||
5 | ** | Exponentiation | right to left | 4**2 |
6 | * | Multiplication | left to right | 2*3 |
/ | Division | 18/9 | ||
% | Remainder | 4%2 | ||
7 | + | Addition | left to right | 2+4 |
– | Subtraction | 4-2 | ||
8 | << | Left shift | left to right | y<<2 |
>> | Right shift | y>>2 | ||
>>> | Unsigned Right shift | y>>>2 | ||
9 | < | Less than | left to right | 3<4 |
<= | Less than or equal | 3<=4 | ||
> | Greater than | 4>3 | ||
>= | Greater than or equal | 4>=3 | ||
in | In | “PI” in MATH | ||
instanceof | Instance of | A instanceof B | ||
10 | == | Equality | left to right | x==y |
!= | Inequality | x!=y | ||
=== | Strictly equal | x===y | ||
!== | Strictly unequal | x!==y | ||
11 | & | Bitwise AND | left to right | x&y |
12 | ^ | Bitwise XOR | left to right | x^y |
13 | | | Bitwise OR | left to right | x|y |
14 | && | Logical AND | left to right | x&&y |
15 | || | Logical OR | left to right | x||y |
16 | ? : | Conditional | right to left | (x>y)?x:y |
17 | Assignment | right to left | x=5 | |
+= | x+=3 | |||
-= | x-=3 | |||
*= | x*=3 | |||
/= | x/=3 | |||
%= | x%=3 | |||
<<= | x<<=2 | |||
>>= | x>>=2 | |||
>>>= | x>>>=2 | |||
&= | x&=y | |||
^= | x^=y | |||
|= | x|=y | |||
18 | yield | Pause function | right to left | yield x |
19 | , | Comma | left to right | x,y |
Understanding operator precedence is essential for writing correct and predictable code. If needed, you can use parentheses to explicitly control the order of evaluation in complex expressions.